How Do I Convert an Existing Callback API to Promises?
Converting an existing callback-based API to use promises in JavaScript involves wrapping the asynchronous functions with a Promise. Here's a step-by-step guide:
Let's assume you have an existing callback-based function like this:
function fetchData(callback) {
// Simulating an asynchronous operation
setTimeout(() => {
const data = { result: 'Some data' };
callback(null, data);
}, 1000);
}
// Example usage
fetchData((error, data) => {
if (error) {
console.error('Error:', error);
} else {
console.log('Data:', data);
}
});
Now, let's convert the fetchData
function to use promises:
function fetchDataPromise() {
return new Promise((resolve, reject) => {
// Simulating an asynchronous operation
setTimeout(() => {
const data = { result: 'Some data' };
// Use resolve to fulfill the promise with the data
resolve(data);
// Use reject to reject the promise with an error
// reject(new Error('Failed to fetch data'));
}, 1000);
});
}
// Example usage with promises
fetchDataPromise()
.then((data) => {
console.log('Data:', data);
})
.catch((error) => {
console.error('Error:', error);
});
In this example:
- The
fetchDataPromise
function returns a new Promise. - Inside the promise constructor, you perform the asynchronous operation.
- If the operation is successful, use
resolve
to fulfill the promise with the result. - If there's an error, use
reject
to reject the promise with an error.
Now, you can use the function with promises, allowing you to use then
and catch
for handling the results and errors.
Converting existing code to promises can make it more readable, manageable, and compatible with modern JavaScript practices. Remember to update the places where the original callback-based function was used to work with the new promise-based version.
-
Using Node.js as a simple web server
Node.js comes with a built-in module called http that can be used to create a simple web server. Below is a basic example of how to create a simple HTTP server using Node.js: const http = require('...
Questions -
Using Node.js require vs. ES6 import/export
Node.js uses CommonJS-style require for module loading, while ES6 introduces a native import and export syntax. Each has its own style and use cases. Using require (CommonJS): // Importing a module...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us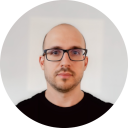
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github