Using Node.js require vs. ES6 import/export
Node.js uses CommonJS-style require
for module loading, while ES6 introduces a native import
and export
syntax. Each has its own style and use cases.
Using require
(CommonJS):
// Importing a module using require
const fs = require('fs');
const path = require('path');
// Exporting from a module using module.exports
const myModule = require('./myModule');
module.exports = myModule;
- Pros:
- Widely used in Node.js and supported in many environments.
- Dynamic loading allows conditionally loading modules.
- Cons:
- Synchronous by default, which can lead to slower startup times.
- Requires additional tools like Babel for using ES6 features in older Node.js versions.
Using import
and export
(ES6 Modules):
// Importing a module using import
import fs from 'fs';
import path from 'path';
// Exporting from a module using export
import myModule from './myModule';
export default myModule;
- Pros:
- Standardized in ECMAScript 6 (ES2015) and later.
- Supports static analysis for better tooling and optimizations.
- Allows for named exports, which makes it clearer which parts of the module are being used.
- Cons:
- Not yet fully supported in all environments (though widely supported in modern Node.js versions).
- Requires a file extension when importing (e.g.,
.js
). - Does not support dynamic loading as
require
does.
Interoperability:
In many modern Node.js projects, you can use a mix of both require
and import
/export
syntax, thanks to tools like Babel or the built-in ESM (ECMAScript Modules) support in recent Node.js versions. For example, you might use import
for newer code and libraries, while still using require
for older or third-party modules.
Migration:
If you are working in a Node.js environment that supports ESM (ECMAScript Modules), you can gradually migrate your codebase from require
to import
/export
. Keep in mind that there might be differences in behavior, especially regarding the handling of circular dependencies and variable hoisting.
Ultimately, the choice between require
and import
/export
depends on your project's requirements, the Node.js version you are targeting, and your personal or team preferences.
-
Is there a map function for objects in Node.js?
In JavaScript, the map function is typically used with arrays to transform each element of the array based on a provided callback function. If you want to achieve a similar result with objects, you...
Questions -
How do you get a list of the names of all files present in a directory in Node.js?
In Node.js, you can use the fs (file system) module to get a list of file names in a directory. Here's an example using the fs.readdir function: const fs = require('fs'); const directoryPath = '/pa...
Questions -
How can I get the full object in Node.js's console.log(), rather than '[Object]'?
By default, console.log() in Node.js will display [Object] when trying to log an object. If you want to see the full contents of an object, you can use util.inspect() from the util module, which is...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us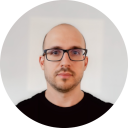
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github