Using Node.js as a simple web server
Node.js comes with a built-in module called http
that can be used to create a simple web server. Below is a basic example of how to create a simple HTTP server using Node.js:
const http = require('http');
// Create a server object
const server = http.createServer((req, res) => {
// Set the response header
res.writeHead(200, {'Content-Type': 'text/plain'});
// Send a response
res.end('Hello, this is a simple Node.js web server!');
});
// Specify the port on which the server will listen
const port = 3000;
// Start the server and listen on the specified port
server.listen(port, () => {
console.log(`Server running at <http://localhost>:${port}/`);
});
In this example:
- We import the
http
module. - We create a server using
http.createServer()
. This function takes a callback function withreq
(request) andres
(response) as parameters. Inside the callback, you handle the incoming request and send the response. - We set the response header using
res.writeHead()
to indicate that the response is plain text. - We send a simple text response using
res.end()
. - We specify the port (in this case, 3000) on which the server will listen.
- We start the server with
server.listen()
.
Save the code in a file, for example, server.js
, and run it using the following command in the terminal:
node server.js
Now, if you open your web browser and navigate to http://localhost:3000/
, you should see the message "Hello, this is a simple Node.js web server!".
This is a very basic example, and in a real-world scenario, you might want to use frameworks like Express.js for more features and better organization of your code. However, for a simple use case, the built-in http
module is sufficient.
-
What is the purpose of Node.js module.exports and how do you use it?
In Node.js, module.exports is a special object that is used to define what a module exports as its public interface. It is used to expose functionality from one module (file) to another module, all...
Questions -
Comparing Node.js Testing Libraries
This guide presents a comparison of top 9 testing libraries for Node.js to help you decide which one to use in your next project
Guides -
How to get `GET` variables in Express.js on Node.js?
In Express.js, you can access GET (query string) variables using the req.query object. The req.query object contains key-value pairs of the query string parameters in a GET request. Here's an examp...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us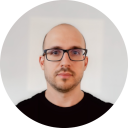
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github