How do I check if a string represents a number in Python?
To check if a string represents a number (float or int) in Python, you can try casting it to a float or int and check if the cast was successful. If the string can't be cast, then it is not a number.
Here is an example:
def is_number(s):
try:
float(s)
return True
except ValueError:
return False
print(is_number('123')) # True
print(is_number('123.456')) # True
print(is_number('123a')) # False
print(is_number('abc')) # False
Alternatively, you can use the isdigit
method of strings:
def is_number(s):
return s.isdigit()
print(is_number('123')) # True
print(is_number('123.456')) # False
print(is_number('123a')) # False
print(is_number('abc')) # False
Note that the isdigit
method will only return True
for strings that consist only of digits and that do not have a decimal point. It will return False
for strings like '123.456'
or '123a'
.
-
How do I pad a string with zeroes in Python?
You can pad a string with zeros in Python by using the zfill() method. This method adds zeros to the left of the string until it reaches the specified length. Here's an example: s = '123' padded = ...
Questions -
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions -
How to check if the string is empty in Python?
To check if a string is empty in Python, you can use the len() function. For example: string = "" if len(string) == 0: print("The string is empty") You can also use the not operator to check if...
Questions -
How do I get a substring of a string in Python?
To get a substring of a string in Python, you can use the string slicing notation, which is string[start:end], where start is the index of the first character of the substring, and end is the index...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us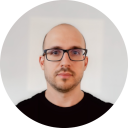
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github