How to catch multiple exceptions in one line (except block)?
To catch multiple exceptions in one except block, you can use the following syntax:
except (SomeException, DifferentException) as e:
pass # handle the exception or pass
If you are using Python 2, you can use the following syntax as the previous one won’t work:
except (SomeException, DifferentException), e:
pass # handle the exception or pass
The last method will still work in Python 2.7 but it is now deprecated in python 3, therefore the first method is recommended.
-
How can I safely create a nested directory in Python?
The most common way to safely create a nested directory in Python is using the pathlib or os modules. Using pathlib You can create a nested directory in python 3.5 or later using the Path and mkdir...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
How to use the ternary conditional operator in Python?
The ternary conditional operator is a shortcut when writing simple conditional statements. If the condition is short and both true and false branches are short too, there is no need to use a multi-...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us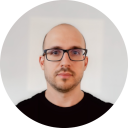
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github