How to Access POST Form Fields in Express
In Express.js, you can access POST form fields using the req.body
object. To do this, you need to use a middleware called body-parser
(for Express versions 4.16.0 and below) or the built-in express.urlencoded()
middleware (for Express versions 4.16.1 and above).
Here's an example using the express.urlencoded()
middleware:
Install
express
(if not already installed) andbody-parser
(if your Express version is 4.16.0 or below):npm install express
Create an Express application:
const express = require('express'); const app = express(); // Middleware to parse urlencoded data app.use(express.urlencoded({ extended: true })); // Route handling POST form submission app.post('/submit-form', (req, res) => { // Access form fields from req.body const username = req.body.username; const password = req.body.password; // Process the form data console.log('Username:', username); console.log('Password:', password); // Send a response res.send('Form submitted successfully!'); }); // Start the server const PORT = 3000; app.listen(PORT, () => { console.log(`Server is listening on port ${PORT}`); });
Create an HTML form:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Form Submission</title> </head> <body> <form action="/submit-form" method="post"> <label for="username">Username:</label> <input type="text" id="username" name="username" required> <label for="password">Password:</label> <input type="password" id="password" name="password" required> <button type="submit">Submit</button> </form> </body> </html>
Submit the form:
- Open the HTML file in a browser.
- Enter values in the form fields and submit the form.
The Express server will receive the POST request, and you can access the form fields using req.body
in the route handler. Make sure to use the express.urlencoded()
middleware to parse the form data. If your Express version is 4.16.1 or above, this middleware is included by default. Otherwise, you might need to install and use the body-parser
middleware as shown in step 1.
-
Using Node.js require vs. ES6 import/export
Node.js uses CommonJS-style require for module loading, while ES6 introduces a native import and export syntax. Each has its own style and use cases. Using require (CommonJS): // Importing a module...
Questions -
Comparing Node.js Testing Libraries
This guide presents a comparison of top 9 testing libraries for Node.js to help you decide which one to use in your next project
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us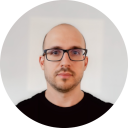
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github