Generate random integers between X and Y in Python?
To generate random integers between x
and y
(inclusive), you can use the random
module in the Python standard library. Here's an example:
import random
x = 1
y = 10
random_number = random.randint(x, y)
print(random_number)
This will generate a random integer between x
and y
, and print it to the console.
You can also use the randrange
function from the random
module to generate a random integer between x
and y
. The difference between randint
and randrange
is that randrange
allows you to specify a step size, whereas randint
does not. For example, to generate a random even integer between x
and y
, you can use the following code:
import random
x = 2
y = 10
step = 2
random_number = random.randrange(x, y+1, step)
print(random_number)
This will generate a random even integer between x
and y
, and print it to the console.
-
How do I pad a string with zeroes in Python?
You can pad a string with zeros in Python by using the zfill() method. This method adds zeros to the left of the string until it reaches the specified length. Here's an example: s = '123' padded = ...
Questions -
How can I randomly select an item from a list in Python?
To randomly select an item from a list in Python, you can use the random.choice() function from the random module. This function takes a list as an argument and returns a randomly selected element ...
Questions -
How do I get the number of elements in a list in Python?
To get the number of elements in a list in Python, you can use the len() function. For example, if you have a list my_list, you can get its length using len(my_list). Here's an example: mylist = [1...
Questions -
How do I check if a string represents a number in Python?
To check if a string represents a number (float or int) in Python, you can try casting it to a float or int and check if the cast was successful. If the string can't be cast, then it is not a numbe...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us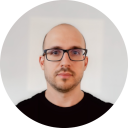
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github