Error: Can't set headers after they are sent to the client
The "Error: Can't set headers after they are sent to the client" error typically occurs in Node.js when you attempt to set HTTP headers (e.g., using res.setHeader()
or res.writeHead()
) after the response has already been sent to the client. This error indicates a logical error in your code where you are trying to modify the response headers after it has already been finalized and sent.
Here are some common causes and solutions for this error:
Asynchronous Operations
If you are performing asynchronous operations and trying to modify the response headers inside a callback, ensure that the response hasn't been sent before you attempt to modify the headers.
app.get('/', (req, res) => {
// Asynchronous operation, e.g., querying a database
someAsyncOperation((data) => {
// Check if response has already been sent
if (!res.headersSent) {
res.setHeader('Content-Type', 'application/json');
res.send(data);
}
});
});
Calling res.send()
Multiple Times
Calling res.send()
or res.end()
more than once in the same request handler can trigger this error. Ensure that you have proper flow control to avoid sending the response multiple times.
app.get('/', (req, res) => {
// Incorrect: sending response twice
res.send('First response');
res.send('Second response'); // This will trigger the error
// Correct: send response only once
res.send('Correct response');
});
Using Middleware Incorrectly
If you are using middleware that modifies the response headers, make sure it is placed in the correct order. Middleware order matters, and you should place middleware that modifies headers before the middleware that sends the response.
Using Both res.setHeader()
and res.send()
If you set headers using res.setHeader()
and also use res.send()
later, consider using the res.set()
method, which combines setting headers and sending the response.
app.get('/', (req, res) => {
res.set('Content-Type', 'application/json');
res.send('{"message": "Hello"}');
});
5. Review Code for Logical Errors:
Carefully review your code for logical errors, especially in control flow, and ensure that you are not unintentionally modifying headers after the response has been sent.
By addressing the above points and carefully examining your code, you should be able to resolve the "Error: Can't set headers after they are sent to the client" issue in your Node.js application.
-
How to check synchronously if file/directory exists in Node.js?
In Node.js, you can use the fs (file system) module to check synchronously if a file or directory exists. The fs.existsSync() function can be used for this purpose. Here's an example: const fs = re...
Questions -
How can I get the full object in Node.js's console.log(), rather than '[Object]'?
By default, console.log() in Node.js will display [Object] when trying to log an object. If you want to see the full contents of an object, you can use util.inspect() from the util module, which is...
Questions -
Job Scheduling in Node.js with BullMQ
This is a comprehensive guide for anyone looking to implement task scheduling with BullMQ in a Node.js application
Guides -
Monitoring Linux with Node Exporter
This guide will teach you how to install and configure Prometheus and Node Exporter for monitoring your Linux servers
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us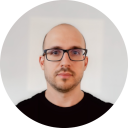
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github