How to check synchronously if file/directory exists in Node.js?
In Node.js, you can use the fs
(file system) module to check synchronously if a file or directory exists. The fs.existsSync()
function can be used for this purpose. Here's an example:
const fs = require('fs');
const pathToFileOrDir = '/path/to/your/file_or_directory';
// Check if the file or directory exists synchronously
if (fs.existsSync(pathToFileOrDir)) {
console.log(`The file or directory at '${pathToFileOrDir}' exists.`);
} else {
console.log(`The file or directory at '${pathToFileOrDir}' does not exist.`);
}
Replace '/path/to/your/file_or_directory'
with the actual path to the file or directory you want to check.
Please note that using synchronous methods, like fs.existsSync()
, can block the event loop, and it is generally recommended to use asynchronous methods to avoid blocking your application. However, in some specific scenarios, synchronous methods might be appropriate.
If you prefer an asynchronous approach, you can use the fs.stat()
function:
const fs = require('fs');
const pathToFileOrDir = '/path/to/your/file_or_directory';
// Check if the file or directory exists asynchronously
fs.stat(pathToFileOrDir, (err, stats) => {
if (err) {
console.log(`The file or directory at '${pathToFileOrDir}' does not exist.`);
} else {
console.log(`The file or directory at '${pathToFileOrDir}' exists.`);
}
});
In the asynchronous example, the fs.stat()
function is used to get information about the file or directory. The presence of an error in the callback indicates that the file or directory does not exist.
-
How do I pass command line arguments to a Node.js program and receive them?
In Node.js, you can pass command line arguments to your script the same as you would for any other command line application. Simply type your arguments after the script path separated with a space ...
Questions -
How can I update Node.js and NPM to their latest versions?
There are several ways to update Node.js to its latest version. Here are three methods: Updating Node.js Using NPM You can use NPM to update Node.js by installing the n package, which will be used ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us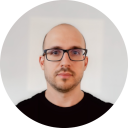
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github