What is the Difference Between a Gauge and a Counter?
Gauges and counters are two core metric types in Prometheus. They serve different purposes and are used to track different kinds of data.
1. Counter
A counter is a metric that only increases over time (or resets to zero). It is used for tracking cumulative values.
Characteristics:
- Monotonically increasing.
- Resets to zero on application restart.
- Cannot decrease.
Common use cases:
- Counting the number of HTTP requests.
- Tracking the total number of errors or failed operations.
- Measuring completed tasks.
Example:
from prometheus_client import Counter
# Define a counter for HTTP requests
http_requests = Counter('http_requests_total', 'Total number of HTTP requests')
# Increment the counter
http_requests.inc() # Increases by 1
http_requests.inc(3) # Increases by 3
PromQL Example:
- Total number of requests:
http_requests_total
- Rate of increase:
rate(http_requests_total[5m])
2. Gauge
A gauge is a metric that can increase or decrease over time. It represents a snapshot of a value at a specific moment.
Characteristics:
- Can go up or down.
- Useful for measuring current states or values.
- Tracks instantaneous values.
Common use cases:
- Monitoring CPU or memory usage.
- Measuring the size of a queue.
- Tracking the number of active connections.
Example:
from prometheus_client import Gauge
# Define a gauge for memory usage
memory_usage = Gauge('memory_usage_bytes', 'Current memory usage in bytes')
# Set the gauge to a specific value
memory_usage.set(500)
# Increment or decrement the gauge
memory_usage.inc(100) # Increases by 100
memory_usage.dec(50) # Decreases by 50
PromQL Example:
- Current memory usage:
memory_usage_bytes
- Average memory usage over 5 minutes:
avg_over_time(memory_usage_bytes[5m])
Key differences at a glance
Feature | Counter | Gauge |
---|---|---|
Behavior | Only increases or resets to zero | Can increase or decrease |
Represents | Cumulative values over time | Instantaneous values |
Use case examples | Total requests, error counts | Memory usage, queue size |
Common operations | Increment | Increment, decrement, set |
-
How to Add Custom HTTP Headers in Prometheus
Here is the content with only the indentation fixed: Adding custom HTTP headers in Prometheus is useful when interacting with a secured remote endpoint, such as when scraping metrics from services ...
Questions -
How To Manage Prometheus Counters
Prometheus counters are metrics that only increase or reset to zero. They are ideal for tracking values like requests, errors, or completed tasks. Managing counters effectively ensures accurate and...
Questions -
How to Monitor Disk Usage in Kubernetes Persistent Volumes
Monitoring disk usage in Kubernetes persistent volumes is crucial for ensuring application stability. Kubernetes does not natively provide metrics for persistent volume usage, but you can use tools...
Questions -
What Is A Bucket In Prometheus?
In Prometheus, a bucket is a concept used in histograms to organize observed values into predefined ranges. Buckets are critical for tracking and analyzing the distribution of values, such as respo...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us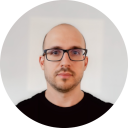
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github