When you build Python applications, you need to manage packages and dependencies. Poetry and Pip are two popular tools that help with this, but they work differently.
Pip is Python's default package installer. It's simple, comes with Python, and focuses on installing packages quickly.
Poetry is a newer tool that handles more than just installation. It manages dependencies, virtual environments, and packaging all in one place.
This article compares these tools to help you choose the best project fit.
What is Pip?
Pip is the standard package installer for Python. Its name stands for "Pip Installs Packages," which describes exactly what it does.
Python has included Pip since version 3.4, so you don't need to install it separately. It replaced an older tool called easy_install
back in 2008 and has been the main way to install Python packages ever since.
Pip does one job and does it well: it gets packages from the Python Package Index (PyPI) and installs them on your system. It's straightforward to use with simple commands, making it perfect for beginners and quick projects.
What is Poetry?
Poetry is a more recent tool created in 2018 by Sébastien Eustace. It's designed to solve common problems that Python developers face when managing projects.
Unlike Pip, Poetry handles many tasks in one tool. It manages your dependencies, creates virtual environments, builds packages, and publishes them to PyPI. Poetry was inspired by package managers from other languages like npm (JavaScript) and Bundler (Ruby).
Poetry's main goal is to make your projects more reliable. It creates detailed lock files that track exact versions of every package you use, ensuring your code works the same way on every computer. This helps eliminate those frustrating "it works on my machine" problems.
Poetry vs. Pip: a quick comparison
Choosing between Poetry and Pip affects how you'll work with Python projects. Each tool has strengths that make it better for different situations.
Here's a simple comparison of their key features:
Feature | Poetry | Pip |
---|---|---|
Main focus | Complete package management | Package installation |
Configuration file | pyproject.toml |
requirements.txt |
Dependency resolution | Advanced, solves conflicts automatically | Basic, installs what you ask for |
Lock files | Built-in (poetry.lock ) |
Needs extra tools (pip-tools) |
Virtual environments | Creates and manages automatically | Needs separate tools (virtualenv) |
Build and publish | Built-in commands | Needs extra tools (setuptools, twine) |
Project creation | Can create new projects | Can't create projects |
Dev dependencies | Separates dev, test, docs dependencies | No built-in separation |
Installation speed | Installs packages in parallel | Installs one at a time by default |
Learning curve | Steeper to learn | Very simple to start using |
Community adoption | Growing quickly | Used everywhere |
Script commands | Can define project commands | Needs other tools (Makefiles) |
Comes with Python | No, separate installation | Yes, included with Python |
Dependency updates | Has commands to update safely | Need to manually edit requirements |
Project configuration
How you define your project's dependencies affects both how easy it is to start working and how well it holds up over time. Pip and Poetry use completely different approaches here.
Pip uses a simple text file called requirements.txt
. You list packages line by line:
# requirements.txt
requests==2.28.1
django>=4.0.0,<5.0.0
numpy~=1.23.0
# For development only
pytest>=7.0.0 # Not separated from production dependencies
flake8>=6.0.0
Pip's approach is simple but has some limitations. There's no built-in way to separate development dependencies from production ones. You don't get automatic lock files to ensure exact versions. You have to handle dependency conflicts manually, and you need separate files for building packages.
For more complex projects, developers often create multiple requirements files:
# requirements/base.txt
requests==2.28.1
django>=4.0.0,<5.0.0
# requirements/dev.txt
-r base.txt
pytest>=7.0.0
flake8>=6.0.0
# requirements/prod.txt
-r base.txt
gunicorn==20.1.0
This approach works, but you need to maintain multiple files and remember how they connect to each other.
Poetry uses a completely different approach with a file called pyproject.toml
. This format organizes everything more clearly:
[tool.poetry]
name = "my-project"
version = "0.1.0"
description = "A sample project"
authors = ["Your Name <your.email@example.com>"]
[tool.poetry.dependencies]
python = "^3.9"
requests = "^2.28.1"
django = "^4.0.0"
numpy = "~1.23.0"
[tool.poetry.group.dev.dependencies]
pytest = "^7.0.0"
flake8 = "^6.0.0"
[build-system]
requires = ["poetry-core>=1.0.0"]
build-backend = "poetry.core.masonry.api"
Poetry's approach has several advantages. It clearly separates project information from dependencies and groups development dependencies separately from production. It works with Python's official packaging standards and automatically creates lock files. You can also define multiple dependency groups for different purposes like development, testing, or documentation.
You can also configure other tools in the same file, keeping all settings in one place:
[tool.pytest]
testpaths = ["tests"]
python_files = "test_*.py"
[tool.black]
line-length = 88
target-version = ['py39']
This means you don't need dozens of different configuration files scattered throughout your project.
Dependency resolution
One of the most significant differences between these tools is how they figure out which versions of packages work together.
Pip uses a simple approach. When you install packages, it processes them one by one in the order you list them:
# Install a package with Pip
pip install requests==2.28.1
# Add another package
pip install numpy==1.23.0
This straightforward approach can cause problems with complex dependencies:
# What happens with conflicting dependencies?
pip install package-a # requires requests>=2.28.0
pip install package-b # requires requests<2.26.0
# The last one installed "wins" - possibly breaking the other package
Pip has improved in recent versions, but still doesn't fully solve complex dependency conflicts. Many developers use an add-on tool called pip-tools to help:
# Using pip-tools for better dependency management
pip install pip-tools
# Create a compiled requirements file with exact versions
pip-compile requirements.in -o requirements.txt
# Install the exact versions
pip install -r requirements.txt
Poetry uses a completely different approach. It analyzes your entire dependency tree before installing anything. This means it checks all packages and their dependencies to make sure everything will work together:
# Adding dependencies with Poetry
poetry add requests@^2.28.1
poetry add numpy@~1.23.0
# Poetry figures out the entire dependency tree at once
# and creates/updates the lock file automatically
When Poetry finds incompatible packages, it doesn't just install the last one you asked for. It tries to find a solution and gives you a clear error when that's not possible:
# Poetry will tell you exactly what's wrong
poetry add incompatible-package
# You might see output like:
# The package incompatible-package requires requests<2.26.0
# but your project requires requests>=2.28.0
After Poetry finds compatible package versions, it saves this exact solution in a lock file called poetry.lock
. This file captures every package version and hash, ensuring your project works exactly the same everywhere:
# Installing from the lock file ensures identical environments
poetry install
# Update dependencies while staying within version constraints
poetry update
This lock file guarantees that your code runs identically on every computer. While pip-tools can create similar lock files, Poetry makes this a standard part of your workflow rather than an extra step.
Virtual environment management
Python projects need virtual environments to keep their packages separate from each other. Pip and Poetry handle this very differently.
Pip doesn't manage virtual environments at all. It just installs packages wherever you point it. You need to use separate tools like venv or virtualenv:
# Traditional workflow with venv and pip
python -m venv myenv
source myenv/bin/activate # On Windows: myenv\Scripts\activate
pip install -r requirements.txt
# Working with the environment
pip list
pip freeze > requirements.txt
deactivate
This approach keeps Pip simple but has drawbacks. You need to remember to activate the right environment before working, and you need to learn multiple tools. Many developers use extra tools like virtualenvwrapper to make this easier.
Poetry handles environments automatically as part of its normal workflow:
# Poetry manages the virtual environment for you
poetry install
# Run commands in the virtual environment
poetry run python script.py
poetry run pytest
# Or start a shell in the environment
poetry shell
By default, Poetry stores environments in a central location on your computer (though you can change this). This approach gives you several benefits. You don't need to remember to activate environments. Your environment stays in sync with your project dependencies. You don't need to learn separate environment tools. And when dependencies change, your environment updates automatically.
If you need more control, Poetry lets you specify which Python version to use:
# Configure Poetry to use a specific Python version
poetry env use python3.10
# List available environments
poetry env list
# Remove environments
poetry env remove python3.9
This integrated approach is especially helpful if you work on multiple projects or have complex dependency requirements. It lets you focus on your code instead of managing Python environments.
Packaging and publishing
When you want to share your Python code with others, you need to package it and publish it to PyPI. The tools handle this process quite differently.
Pip doesn't help with packaging or publishing at all. Instead, you need to use multiple other tools:
# Traditional packaging workflow
pip install setuptools wheel twine
# Create a setup.py file (requires manual configuration)
# Build the package
python setup.py sdist bdist_wheel
# Upload to PyPI
twine upload dist/*
This approach requires you to know several different tools and create multiple configuration files. You need a setup.py
file for package details and dependencies. You need a MANIFEST.in
file for including non-Python files. And you need a .pypirc
file for repository settings.
Poetry simplifies this entire process with built-in commands that handle everything for you:
# Publishing with Poetry
poetry build
poetry publish
# Or do both in one step
poetry publish --build
Poetry gets all the information it needs from your pyproject.toml
file, so you don't need to duplicate it elsewhere. It handles creating packages and uploading them to PyPI:
# Publish to a custom repository
poetry publish --repository my-repository
# Build specific formats
poetry build --format wheel
Poetry follows modern packaging standards (PEP 517 and PEP 518), making it easier for people who publish packages regularly.
Script and command management
Most Python projects need commands to run tests, start servers, or perform other tasks. Pip and Poetry handle this differently as well.
Pip doesn't include any way to define project commands. Developers typically use other approaches:
# In setup.py (if using setuptools)
setup(
# ...
entry_points={
'console_scripts': [
'my-command=my_package.module:function',
],
},
)
Many projects use Makefiles or shell scripts instead:
# Makefile approach
.PHONY: test
test:
pytest tests/
.PHONY: lint
lint:
flake8 my_package/
This means each project might use different ways to define commands, making it harder to learn new projects.
Poetry lets you define commands directly in your project configuration:
[tool.poetry.scripts]
my-command = "my_package.module:function"
start-server = "my_package.server:main"
These commands become available through Poetry's run command or after installing the package:
# Run a defined script
poetry run my-command
# Or after installing the package
pip install -e .
my-command
Poetry also lets you run any command in your project's virtual environment without activating it first:
# Run arbitrary commands in the virtual environment
poetry run pytest --cov=my_package
poetry run flask run --debug
This approach creates consistency across projects using Poetry, makes commands easy to discover, and ensures they run in the right environment.
Development workflow
The day-to-day experience of using these tools shows their most significant differences. Let's look at how you'd work with each one.
A typical Pip workflow involves multiple steps and tools:
# Typical Pip workflow
python -m venv venv
source venv/bin/activate
pip install -r requirements.txt
pip install -r requirements-dev.txt
# After adding new dependencies
pip freeze > requirements.txt
# Updating dependencies
pip install -U package-name
pip freeze > requirements.txt
This approach is familiar but has several drawbacks. You must remember to activate the environment. You have to update requirements files manually. There's no standard way to separate dependency groups. Updating specific dependencies is tedious. And creating reproducible environments is difficult.
Poetry provides a more streamlined workflow:
# Poetry workflow
poetry install
# Add new dependencies
poetry add requests
poetry add pytest --group dev
# Update dependencies
poetry update
poetry update requests
# Install only production dependencies
poetry install --without dev
Poetry's workflow offers several advantages. Environments are managed automatically. Dependencies are grouped by purpose. Everyday tasks have straightforward commands. The lock file is updated automatically. And installations are identical across environments. These benefits are especially valuable for teams and larger projects, where consistency helps everyone work together more smoothly.
Final thoughts
Poetry is ideal for modern Python projects with complex dependencies and frequent package publishing, making it great for team projects.
On the other hand, Pip is perfect for simpler projects, learning Python, and legacy projects using requirements.txt files.
Both tools have their strengths, with many developers using Poetry for serious development and Pip for quick scripts. Your choice depends on your project needs and preferences.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us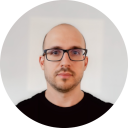
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github