pipenv is a tool that combines package management and virtual environments into a straightforward interface. It does the job of both pip
and virtualenv
, making Python development faster and easier.
This article will show you how to use pipenv
, highlight its best features, and teach you how to make your Python development smoother.
Prerequisites
Before you start with pipenv
, make sure you have Python installed on your computer. pipenv
works with Python 3.7 or newer and needs at least one Python version on your system.
Why use pipenv
?
pipenv
makes Python development better in several important ways:
It combines
pip
andvirtualenv
into a single tool, so you don't need to switch between them.It creates a
Pipfile.lock
that tracks exact package versions, so your code works the same way on every computer.It automatically creates and manages virtual environments for you, saving you from typing multiple commands.
It resolves package dependencies smartly, helping you avoid package conflicts.
Think of pipenv
as a power tool that replaces your separate tools with one that's easier to use. It simplifies how you install packages and manage environments.
In this guide, you'll learn how pipenv
can make your Python projects more reliable and easier to manage.
Installing pipenv
Now that you know what pipenv
is and why it's useful, let's get it set up on your machine.
pipenv
isn't included with Python by default, so you'll need to install it manually. The good news is it's simple to do. You'll use pip
, Python’s package installer, to get it.
Before you begin, make sure Python is already installed by running:
python3 --version
You should see something like:
Python 3.13.2
Now install pipenv
using pip:
pip install --user pipenv
The --user
flag installs it just for your user account, avoiding system-wide permission issues.
For other installation methods (like using Homebrew on macOS or package managers on Linux), check the official Pipenv installation guide.
To make sure it worked, check the version:
pipenv --version
pipenv, version 2024.4.1
Great! Now pipenv
is ready to use.
Getting started with pipenv
Now let's see how pipenv
works. It makes Python development easier by handling packages and virtual environments together.
First, create a folder for your project:
mkdir pipenv-demo && cd pipenv-demo
With pipenv
, you can create an environment and install packages in one step:
pipenv install requests
This single command does four things at once:
- Creates a virtual environment just for this project
- Makes a Pipfile
that lists your project's packages
- Creates a Pipfile.lock
that saves exact package versions
- Installs the requests
package
When you run the command, you'll see output similar to this (with colored text in the terminal to highlight key steps):
To start using this environment, type:
pipenv shell
Launching subshell in virtual environment...
source /Users/username/.local/share/virtualenvs/pipenv-demo-7sw_cn0q/bin/activate
stanley@MacBookPro pipenv-demo % source /Users/username/.local/share/virtualenvs/pipenv-demo-7sw_cn0q/bin
/activate
You're now inside your project's environment. Your prompt might look different to show this.
You can install multiple packages at once:
pipenv install numpy pandas matplotlib
Or specify a minimum version:
pipenv install 'django>=4.2'
Let's test your setup with a simple script. Create a file called app.py
:
import requests
import numpy as np
# Make a simple HTTP request
response = requests.get("https://httpbin.org/json")
data = response.json()
# Create a sample array
arr = np.array([1, 2, 3, 4, 5])
print(f"Request status: {response.status_code}")
print(f"Array mean: {np.mean(arr)}")
Run it:
python app.py
Request status: 200
Array mean: 3.0
If you don't want to activate the shell first, just use:
pipenv run python app.py
This runs your script in the right environment automatically.
You can see how pipenv
simplifies Python by handling packages and environments in one tool. It's much easier than juggling separate tools like pip
and virtualenv
.
Managing dependencies with pipenv
Let's examine how pipenv
helps you manage packages throughout your project.
When you run pipenv install
, it creates two important files:
Pipfile
: A simple file that lists your project's packagesPipfile.lock
: A detailed file that locks the exact versions of everything
These files ensure your project works the same way on any computer. Let's look at the Pipfile
:
cat Pipfile
[[source]]
url = "https://pypi.org/simple"
verify_ssl = true
name = "pypi"
[packages]
requests = "*"
numpy = "*"
pandas = "*"
matplotlib = "*"
[dev-packages]
[requires]
python_version = "3.13"
The Pipfile
is easy to read. The *
means any version of the package is fine.
The Pipfile.lock
file contains much more detail:
cat Pipfile.lock | head -20
{
"_meta": {
"hash": {
"sha256": "5ca65f6b3a19b9f19115cea085a20b39583d46025a3dd889fb6e7ba84d8519c9"
},
"pipfile-spec": 6,
"requires": {
"python_version": "3.13"
},
"sources": [
{
"name": "pypi",
"url": "https://pypi.org/simple",
"verify_ssl": true
}
]
},
"default": {
"certifi": {
"hashes": [
This file stores the exact version of every package and sub-package. Never edit this file by hand.
To update all your packages to their latest versions:
pipenv update
To update just one package:
pipenv update requests
Want to see how packages depend on each other? Use:
pipenv graph
matplotlib==3.10.1
├── contourpy
│ └── numpy
├── cycler
├── fonttools
...
requests==2.32.3
├── certifi
├── charset-normalizer
├── idna
└── urllib3
This shows you which packages depend on others.
To remove a package you don't need anymore:
pipenv uninstall pandas
This removes the package and updates both files.
Working on a team? When you download the project, just run:
pipenv install
This installs all packages at their exact locked versions, so everyone's environment matches perfectly.
For deploying to servers, use:
pipenv install --deploy
This makes sure everything matches exactly what's in the lock file.
With these commands, pipenv
helps you keep your project consistent whether you're developing locally or deploying to production.
Development vs. production dependencies
Most Python projects need two types of packages: ones that your app needs to run (production packages) and ones that only developers need (development packages). pipenv
makes it easy to keep these separate.
This separation gives you three significant benefits:
- Your production app stays small and only has what it really needs
- All developers use the same tools
- Deployment is simpler because you know exactly what belongs in production
When you run pipenv install package_name
, it gets added as a production package. For tools you only need during development (like testing or code formatting), add the --dev
flag:
pipenv install --dev pytest black mypy
This puts these packages in a separate [dev-packages]
section:
cat Pipfile
...
[packages]
numpy = "*"
matplotlib = "*"
requests = "*"
[dev-packages]
pytest = "*"
black = "*"
mypy = "*"
[requires]
python_version = "3.13"
When you deploy to production, install only what your app needs to run:
pipenv install --deploy --ignore-dev
This keeps your production environment clean.
To use development tools with your project, run them through pipenv
:
pipenv run pytest
pipenv run black .
This runs the tools in your project's environment with access to all your packages.
For projects with complex testing needs, create shortcuts by adding a script:
pipenv install --dev pytest pytest-cov
Then edit your Pipfile
to add:
...
[scripts]
test = "pytest --cov=mypackage"
Now you can run tests with one short command:
pipenv run test
This creates standard commands that everyone on your team can use.
Keeping development and production packages separate keeps your project clean and organized, even as it grows larger.
Final thoughts
This article explored the key features of pipenv
to help you modernize your Python workflow. With pipenv
, you can manage packages, virtual environments, and project dependencies with a single, efficient tool.
To explore the tool in detail, check out the official documentation.
Thanks for reading!
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us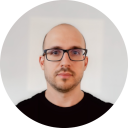
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github