Python offers developers a rich ecosystem of web frameworks, Python's web development ecosystem provides a diverse range of frameworks to match different project requirements and development styles.
Among the most prominent options are Django and FastAPI – two frameworks representing fundamentally different application design and implementation philosophies.
This article compares Django and FastAPI across multiple dimensions to help you determine which one better suits your project requirements.
What is Django?
Django is a full-featured Python web framework released in 2005. Originally built for news websites, it has become the foundation for major platforms like Instagram and Pinterest.
Django provides everything needed to develop web applications without relying on third-party tools. It has an integrated ORM, an auto-generated admin interface, a complete authentication system, a templating engine, and built-in security protections.
What is FastAPI?
FastAPI is a modern, high-performance Python framework that was launched in 2018 and built specifically for API development. Unlike Django, which provides a full-stack solution, FastAPI focuses on building fast, scalable web services with minimal overhead.
It is built on Starlette for asynchronous request handling and Pydantic for automatic validation and data serialization. FastAPI ensures automatic validation, documentation, and editor support by leveraging Python's type hints. It supports async/await
natively, making it highly efficient for handling concurrent requests.
Philosophy and Design Approach
Django was created with the mantra "for perfectionists with deadlines," focusing on developer productivity through convention over configuration. Its design follows these core principles:
- Completeness: Django includes solutions for nearly every aspect of web development including ORM, templating, forms, authentication, admin interface, and more.
- DRY (Don't Repeat Yourself): The framework encourages code reuse and maintains consistency across components.
- Explicit over implicit: Django prefers clear, readable code over "magic" or hidden functionality.
- Loose coupling: Components are independent, allowing you to use only what you need.
Django's architecture is built around a Model-View-Template (MVT) pattern, a variation of the traditional MVC pattern, promoting a clear separation of concerns.
FastAPI takes a very different approach, focusing on performance, simplicity, and modern Python features:
- Performance first: Built on Starlette and Pydantic, FastAPI delivers exceptional speed, rivaling Node.js and Go in benchmarks.
- Type safety: The framework fully embraces Python's type hints, using them for validation, documentation, and editor support.
- Standards-based: FastAPI adheres to OpenAPI and JSON Schema standards, enabling automatic documentation and client generation.
- Incremental adoption: You can use as little or as much of FastAPI as needed, making it highly adaptable.
Unlike Django's full-stack nature, FastAPI is primarily an API framework, designed to build high-performance web services rather than complete web applications with server-rendered templates.
Setup and project structure
The initial setup experience reveals fundamental differences in how these frameworks approach development.
In Django, setting up a minimal project requires several steps:
Install Django:
pip install django
Create a project:
django-admin startproject hello_world
cd hello_world
Create an app:
python manage.py startapp core
Then you need to define a view, configure URLs, and modify settings:
from django.http import HttpResponse
def hello(request):
return HttpResponse("Hello, World!")
# hello_world/urls.py
from django.urls import path
from core.views import hello
urlpatterns = [
path('', hello, name='hello'),
]
# Add 'core' to INSTALLED_APPS in settings.py
Finally, run the development server:
python manage.py runserver
In contrast, FastAPI provides a much more streamlined experience.
You install FastAPI and ASGI server:
pip install fastapi uvicorn[standard]
Create a single file (main.py
):
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def hello():
return {"message": "Hello, World!"}
Run the application:
uvicorn main:app --reload
FastAPI's approach requires significantly less boilerplate and configuration, making it quicker to set up simple applications. However, Django's structure becomes valuable as applications grow more complex, providing clear organization patterns.
Data handling and validation
One of the most significant differences between these frameworks is how they handle data validation and database interactions.
Django's ORM (Object-Relational Mapper) is one of its strongest features, providing a powerful abstraction over SQL databases:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
description = models.TextField(blank=True)
price = models.DecimalField(max_digits=10, decimal_places=2)
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.name
For input validation, Django uses Form
classes:
from django import forms
from .models import Product
class ProductForm(forms.ModelForm):
class Meta:
model = Product
fields = ['name', 'description', 'price']
def clean_price(self):
price = self.cleaned_data['price']
if price <= 0:
raise forms.ValidationError("Price must be greater than zero")
return price
These forms can be used for data validation and HTML form generation, though they become somewhat cumbersome in API contexts.
FastAPI takes a radically different approach, using Pydantic models for validation:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel, Field, validator
from typing import Optional
from decimal import Decimal
app = FastAPI()
class ProductCreate(BaseModel):
name: str
description: Optional[str] = None
price: Decimal = Field(..., gt=0)
@validator('name')
def name_must_not_be_empty(cls, v):
if not v.strip():
raise ValueError('Name cannot be empty')
return v
@app.post("/products/")
async def create_product(product: ProductCreate):
# The product is already validated by Pydantic
# Here you would typically save to database
return {"id": 1, **product.dict()}
FastAPI's validation is integrated directly into route definitions, making it more concise for API development. It handles complex validation scenarios including nested models, lists, and custom validators.
FastAPI doesn't include an ORM for database access, leaving you free to choose solutions like SQLAlchemy, Tortoise ORM, or even Django's ORM.
A common approach is to use SQLAlchemy with async support:
from sqlalchemy.ext.asyncio import create_async_engine, AsyncSession
from sqlalchemy.orm import sessionmaker, declarative_base
from sqlalchemy import Column, Integer, String, Numeric
Base = declarative_base()
class ProductModel(Base):
__tablename__ = "products"
id = Column(Integer, primary_key=True)
name = Column(String, nullable=False)
description = Column(String, nullable=True)
price = Column(Numeric(10, 2), nullable=False)
# Database connection and session
engine = create_async_engine("postgresql+asyncpg://user:password@localhost/db")
async_session = sessionmaker(engine, class_=AsyncSession, expire_on_commit=False)
# In the route handler
@app.post("/products/")
async def create_product(product: ProductCreate):
db_product = ProductModel(**product.dict())
async with async_session() as session:
session.add(db_product)
await session.commit()
await session.refresh(db_product)
return db_product
Django's approach provides a more integrated solution with less configuration, while FastAPI offers greater flexibility and native async database support, albeit with more setup required.
API features and documentation capabilities
Django and FastAPI approach API development and documentation differently. Django relies on the Django REST Framework (DRF), an external package that adds API functionality, while FastAPI includes built-in support for API generation and documentation.
Django itself does not provide API capabilities, so you use DRF to build and manage APIs. DRF introduces serialization, viewsets, and automatic routing but requires additional setup.
# serializers.py
from rest_framework import serializers
from .models import Product
class ProductSerializer(serializers.ModelSerializer):
class Meta:
model = Product
fields = ['id', 'name', 'description', 'price', 'created_at']
# views.py
from rest_framework import viewsets
from .models import Product
from .serializers import ProductSerializer
class ProductViewSet(viewsets.ModelViewSet):
queryset = Product.objects.all()
serializer_class = ProductSerializer
This setup is feature-rich and widely used but requires installing DRF, defining serializers separately from models, configuring viewsets, and setting up documentation tools like drf-yasg or Spectacular.
FastAPI is designed for API development and integrates validation and documentation directly into the framework.
from fastapi import FastAPI, HTTPException, Path
from pydantic import BaseModel
from typing import List, Optional
app = FastAPI(
title="Product API",
description="API for managing products",
version="1.0.0"
)
class Product(BaseModel):
id: int
name: str
description: Optional[str] = None
price: float
class Config:
schema_extra = {
"example": {
"id": 1,
"name": "Smartphone",
"description": "Latest model with great features",
"price": 799.99
}
}
@app.get("/products/", response_model=List[Product], tags=["products"])
async def get_products():
return db_products # Placeholder for actual database query
@app.get(
"/products/{product_id}",
response_model=Product,
responses={404: {"description": "Product not found"}},
tags=["products"]
)
async def get_product(
product_id: int = Path(..., gt=0, description="The ID of the product to retrieve")
):
if product_id not in db_products:
raise HTTPException(status_code=404, detail="Product not found")
return db_products[product_id]
FastAPI automatically generates:
- Interactive documentation with Swagger UI (at
/docs
) - Alternative documentation with ReDoc (at
/redoc
) - OpenAPI schema (at
/openapi.json
) - Client library code generators
Development speed and ecosystem maturity
The frameworks differ significantly in development velocity and ecosystem support.
Django accelerates development through its built-in admin interface, which provides immediate content management and automatic form generation from models.
It also includes a complete authentication system, built-in user management with permissions, an integrated testing framework, management commands for common operations, and a signals system for decoupled components.
The Django ecosystem encompasses thousands of packages for virtually any use case, from content management to e-commerce and geospatial applications. Its long history has created a comprehensive collection of solutions, tutorials, and community resources.
Django's established conventions also standardize project structure:
myproject/
├── manage.py
├── myproject/
│ ├── __init__.py
│ ├── settings.py
│ ├── urls.py
│ └── wsgi.py
└── myapp/
├── __init__.py
├── admin.py
├── apps.py
├── migrations/
├── models.py
├── tests.py
└── views.py
This standardization makes onboarding new developers more straightforward, as most Django developers understand this structure.
FastAPI optimizes API development speed by leveraging automatic validation from type hints and built-in documentation generation.
It also supports dependency injection for modular code, reduces boilerplate, and directly maps route handlers to endpoints. While newer, FastAPI's ecosystem is growing rapidly, with packages for authentication, database integration, and deployment.
It benefits from compatibility with the broader Python async ecosystem, including libraries for databases, messaging, and caching.
FastAPI projects typically have more flexible structures, with a common pattern looking like:
myproject/
├── main.py
├── requirements.txt
├── alembic/
│ └── ... (database migrations)
├── app/
│ ├── __init__.py
│ ├── dependencies.py
│ ├── models/
│ ├── routers/
│ ├── schemas/
│ └── services/
└── tests/
This flexibility allows tailoring the structure to specific project needs but requires more upfront architecture decisions.
Final thoughts
Django and FastAPI take different approaches to web development. Django is a full-stack framework designed for rapid development, offering a mature ecosystem, built-in admin interface, and integrated components for minimal setup.
FastAPI focuses on high-performance API development, with async support, automatic documentation, and strong type safety, making it ideal for microservices and high-throughput applications.
The best choice depends on project requirements, team expertise, and performance needs. Both frameworks continue to evolve, ensuring Python remains a powerful option for web development.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us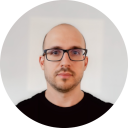
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github