Playwright vs Selenium: The Definitive Comparison
A smooth and engaging user experience is the key to success in today's competitive software landscape. This is where end-to-end (E2E) testing comes in, simulating real-world user interactions and testing how different parts of your application work together.
This guide compares two powerful E2E testing tools: Playwright and Selenium to see which one you should adopt in 2025. Let's get started!
What is Playwright?
Playwright is a modern testing framework developed by Microsoft. Released in 2020, it quickly gained popularity with over 61,000 GitHub stars and 4 million weekly NPM downloads. This rapid adoption demonstrates the industry's recognition of Playwright's capabilities and reliability.
Playwright operates through the DevTools Protocol, which enables direct communication with browsers at a low level. This approach allows for more precise control over browser behavior and enables advanced features like network interception, geolocation mocking, and permission handling.
It supports all major browser engines (Chromium, WebKit, and Firefox) across Windows, Linux, and macOS, ensuring comprehensive coverage of user environments.
What is Selenium?
Selenium is one of the oldest testing frameworks, introduced in 2004. With nearly two decades of development and community contributions, it has established itself as an industry standard for browser automation.
This open-source suite supports scripting in various languages (Java, Python, C#, Ruby, JavaScript, etc.) and all major browsers, making it versatile for diverse development teams.
Selenium's suite includes four main components:
- Selenium IDE: A browser extension that records user interactions and generates scripts, ideal for test prototyping.
- Selenium RC (Remote Control): The original tool for simulating interactions across browsers (largely superseded by WebDriver).
- Selenium WebDriver: The core component that enables direct communication with browsers using their native automation interfaces.
- Selenium Grid: A server that allows parallel test execution on multiple machines and browser configurations, essential for large-scale testing.
Let's explore how these powerful tools compare across key evaluation criteria.
1. Ease of installation
Playwright provides an exceptionally streamlined installation experience. It requires only the relevant programming language environment (e.g., Node.js for JavaScript implementations).
A single command like installs Playwright and automatically downloads all
necessary browsers. It also provides a sample test in the tests
directory and
automatically creates a Playwright configuration file.
This "batteries included" approach means you can start writing and running tests within minutes of installation.
npm init playwright@latest
After running this command, Playwright guides you through a setup wizard that helps configure your testing environment according to your preferences, including selecting browsers and test runners.
Selenium, while having improved its installation process significantly with version 4.6.0, still requires more manual configuration. The Selenium Manager introduced in this version automatically handles the downloading of browsers and drivers when you install Selenium using your programming language's package manager.
However, unlike Playwright, Selenium does not automatically create the directory structure or provide sample tests. You'll need to manually set up your project structure, install appropriate language bindings, and configure your test environment.
2. Learning curve and language support
Playwright features an intuitive API designed with modern development practices in mind. Its documentation is comprehensive, covering advanced capabilities like support for web components, shadow DOM, and iframes with clear examples.
Playwright's code completion and intelligent suggestions in IDEs like Visual Studio Code further flatten the learning curve. It supports multiple programming languages including JavaScript/TypeScript, Python, .NET, and Java, accommodating diverse development teams.
# Playwright Python example
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto("https://example.com")
print(page.title())
browser.close()
Selenium has stood the test of time with its straightforward API and extensive documentation augmented by countless community resources, tutorials, and Stack Overflow discussions.
Its most significant advantage is its unparalleled language support, including C#, Python, Ruby, Kotlin, JavaScript, Java, and more. This extensive language compatibility makes Selenium an excellent choice for polyglot organizations with varied technology stacks.
# Selenium Python example
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com")
print(driver.title)
driver.quit()
For new projects without language constraints, Playwright's modern API design may provide a gentler learning curve. However, in environments with existing expertise in a specific language that Playwright doesn't support, Selenium remains the more accessible option.
3. Debugging tools
Playwright excels with a comprehensive suite of debugging tools:
- VSCode Debugger Integration: Enables direct test step-through in the popular code editor.
- Playwright Inspector: A graphical interface that allows step-by-step test execution, live locator editing, and actionability log viewing.
- Trace Viewer: A sophisticated tool for examining recorded traces of test executions, including screenshots, DOM snapshots, and network requests.
- Browser Developer Tools: Running Playwright in debug mode exposes the browser's native developer tools.
- Verbose API Logs: Detailed logging of all API interactions when the DEBUG environment variable is set.
- Headed Mode with Slow-Motion: Tests can run visually with configurable execution speed for easier observation.
These tools work together to provide a multi-dimensional view of test execution, making it easier to identify issues ranging from timing problems to selector failures.
// Trace recording in Playwright
await page.goto('https://example.com');
await page.screenshot({ path: 'screenshot.png' });
await context.tracing.stop({ path: 'trace.zip' });
// This trace file can be opened in the Playwright Trace Viewer
Selenium offers more limited debugging capabilities, primarily relying on:
- Logging: Various levels of verbosity for tracking execution
- Screenshots: Capturing browser state at specific points
- IDE Integration: Standard debugging techniques like breakpoints
While these tools can be effective, they lack the integrated, purpose-built debugging experience that Playwright provides. Selenium users often need to supplement these basic capabilities with third-party tools or custom logging solutions.
// Basic debugging in Selenium
// Taking a screenshot
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
Files.copy(screenshot.toPath(), Paths.get("screenshot.png"));
// Setting up logging
System.setProperty("webdriver.chrome.verboseLogging", "true");
For complex applications or intricate test scenarios, Playwright's sophisticated debugging toolkit can significantly reduce troubleshooting time and effort.
4. Browser support
Playwright offers exceptional cross-browser support, covering all major rendering engines:
- Chromium (including Chrome and Edge): Full support with advanced capabilities.
- Firefox: Complete support with consistent API across browsers.
- WebKit (Safari): Native support without requiring additional setup.
Additionally, Playwright provides robust mobile viewport emulation for testing responsive designs and mobile user experiences. It offers granular control over browser versions and updates, allowing tests to run against specific browser versions as needed. This flexibility is valuable for applications that must support particular browser environments.
Selenium supports an even wider range of browsers, including older ones like Internet Explorer, which may be necessary for enterprise applications with legacy support requirements. It integrates well with browser-specific drivers like ChromeDriver, GeckoDriver, and SafariDriver. For mobile testing, Selenium works with Appium to test on real mobile devices and emulators, though this requires additional setup.
Both tools provide solid cross-browser testing capabilities, but Playwright's unified API across browsers simplifies test maintenance, while Selenium's support for legacy browsers may be critical for applications with specific compatibility requirements.
5. Auto waiting
Auto-waiting mechanisms ensure that actions are performed only when elements are ready for interaction, significantly reducing flaky tests caused by timing issues.
Playwright implements sophisticated auto-waiting through its locator methods
like getByRole()
, page.getByLabel()
, and page.getByAltText()
. Before
performing actions, Playwright automatically:
- Checks element visibility.
- Verifies element stability (not moving).
- Confirms the element is enabled.
- Ensures the element is editable (for input-related actions).
If these conditions aren't met within a configurable timeout, Playwright aborts the action with a descriptive error message. This comprehensive approach eliminates most timing-related failures without requiring explicit waits in test code.
// Playwright automatically waits for the button to be visible, stable, and enabled
await page.getByRole('button', { name: 'Submit' }).click();
Selenium lacks built-in auto-waiting mechanisms, instead relying on:
- Implicit waits: Global settings that define how long WebDriver should wait for elements.
- Explicit waits: Specific conditions that must be met before proceeding.
These approaches require manual configuration and explicit code in test scripts, leading to either unnecessary waiting time or potential race conditions.
// Selenium requires explicit waits to avoid timing issues
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement button = wait.until(ExpectedConditions.elementToBeClickable(By.id("submit")));
button.click();
Playwright's automatic handling of element actionability significantly reduces the code needed to create stable tests and minimizes the expertise required to write timing-resilient test scripts.
6. Retries
Test retry capabilities help distinguish between genuine bugs and intermittent failures caused by environmental factors, network issues, or timing problems.
Playwright offers comprehensive retry functionality:
- Locator retries: Built into methods like
page.getByRole()
, which automatically retry finding elements - Global retry configuration: Set maximum retry attempts for all tests
- Test-specific overrides: Configure specific retry settings for individual tests or test groups
- Detailed reporting: Tests are categorized as "passed" (successful initially), "flaky" (fails initially but succeeds on retry), or "failed" (never passes)
This multi-layered approach allows teams to identify and address flaky tests while preventing them from blocking CI/CD pipelines.
// Playwright test retry configuration
// In playwright.config.js
module.exports = {
retries: 3, // Retry failed tests up to 3 times
};
// For a specific test
test('my flaky test', { retries: 5 }, async ({ page }) => {
// This test will retry up to 5 times
});
Selenium does not provide built-in retry mechanisms for tests. Implementing retry logic requires custom code or integration with test frameworks that support retries, such as TestNG or JUnit. This limitation can complicate efforts to manage flaky tests effectively.
// Example of custom retry logic needed with Selenium
@Test(retryAnalyzer = RetryAnalyzer.class)
public void flakyTest() {
// Test implementation
}
// Requires a custom RetryAnalyzer implementation
public class RetryAnalyzer implements IRetryAnalyzer {
private int count = 0;
private static final int MAX_RETRY = 3;
@Override
public boolean retry(ITestResult result) {
if (!result.isSuccess()) {
if (count < MAX_RETRY) {
count++;
return true;
}
}
return false;
}
}
Playwright's built-in retry capabilities significantly reduce the engineering effort required to manage test flakiness, particularly in large test suites running in CI/CD environments.
7. Multiple tabs
Support for multiple tabs or windows is essential for testing complex user journeys that span across different browser contexts.
Playwright provides elegant handling of multiple tabs within browser contexts:
// Playwright multiple tab handling
const context = await browser.newContext();
// Create two pages within the same context
const pageOne = await context.newPage();
const pageTwo = await context.newPage();
await pageOne.goto('https://example.com');
await pageTwo.goto('https://another-example.com');
// Interact with both pages
await pageOne.getByRole('button', { name: 'Login' }).click();
await pageTwo.getByRole('link', { name: 'Register' }).click();
// Retrieve all pages of a browser context
const allPages = context.pages();
This approach makes it straightforward to create and manage multiple tabs that share the same session state, cookies, and local storage—ideal for testing features like "open in new tab" functionality.
Selenium also supports multiple tabs/windows, though with a slightly more complex API:
// Selenium multiple tab handling
// Opens a new tab and switches to it
String originalWindow = driver.getWindowHandle();
driver.switchTo().newWindow(WindowType.TAB);
driver.get("https://example.com");
// Opens a new window
driver.switchTo().newWindow(WindowType.WINDOW);
driver.get("https://another-example.com");
// Switch back to the original tab
driver.switchTo().window(originalWindow);
// Get handles for all open tabs/windows
Set<String> allWindows = driver.getWindowHandles();
Both tools effectively support multiple tabs, but Playwright's context-based approach provides a more intuitive API and better isolation between different browser states.
8. Test isolation
Test isolation ensures that each test runs in a clean environment, preventing tests from affecting each other and making test failures more reproducible.
Playwright excels in test isolation through its BrowserContext concept:
// Playwright test isolation
test('first test', async ({ browser }) => {
// This creates a fresh context for this test only
const context = await browser.newContext();
const page = await context.newPage();
// Any cookies, localStorage, etc. are isolated to this context
await page.goto('https://example.com');
await context.close();
});
test('second test', async ({ browser }) => {
// This test gets its own fresh context
const context = await browser.newContext();
const page = await context.newPage();
// No shared state with the previous test
await page.goto('https://example.com');
await context.close();
});
These contexts function like separate user profiles or incognito windows, but they're much lighter and faster to create. Each test gets a pristine environment with fresh cookies, local storage, session storage, and cache, preventing cross-test contamination.
Selenium does not provide built-in mechanisms for test isolation. While it's possible to clear cookies and local storage between tests, this requires manual implementation:
// Manual test isolation in Selenium
@BeforeEach
public void setUp() {
driver = new ChromeDriver();
}
@AfterEach
public void tearDown() {
// Must manually clean up state between tests
driver.manage().deleteAllCookies();
// Clear localStorage
((JavascriptExecutor) driver).executeScript("window.localStorage.clear();");
driver.quit();
}
This approach is more error-prone and potentially slower than Playwright's built-in isolation. For large test suites where test independence is critical, Playwright's automatic isolation provides significant benefits in reliability and maintainability.
9. Scalability
Scalability determines how effectively a testing framework can handle large test suites and distribute test execution across computing resources.
Playwright offers excellent scalability features:
- Automatic parallelization: Runs test files in parallel across available CPU cores by spawning worker processes.
- Fine-grained control: Allows parallelizing tests within a single file if needed.
- Test sharding: Supports distributing tests across multiple machines to reduce execution time.
- Worker isolation: Each test worker runs in isolation to prevent interference.
// Playwright parallelization configuration
// In playwright.config.js
module.exports = {
workers: 8, // Run tests in 8 parallel workers
// Alternatively, use a percentage of CPU cores
// workers: '50%',
};
For distributed execution across multiple machines:
# On machine 1
npx playwright test --shard=1/3
# On machine 2
npx playwright test --shard=2/3
# On machine 3
npx playwright test --shard=3/3
Selenium scales through Selenium Grid, a server that distributes test execution across multiple machines:
// Selenium Grid example
WebDriver driver = new RemoteWebDriver(
new URL("http://grid-server:4444"),
new ChromeOptions()
);
Selenium Grid supports:
- Running tests against different browser/OS combinations.
- Load balancing across multiple nodes.
- Parallel test execution to reduce overall test time.
Both tools provide robust solutions for test scaling, with Playwright offering simpler configuration for local parallelization and Selenium Grid providing a mature solution for distributed testing across heterogeneous environments.
10. CI/CD integration
Playwright provides exceptional CI/CD support with detailed documentation and sample configurations for major providers:
- GitHub Actions
- Azure Pipelines
- CircleCI
- Jenkins
- GitLab CI
These configurations include features like:
- Parallel test execution
- Report generation and merging
- Artifact storage for screenshots and videos
- Cache optimization for faster runs
# Example GitHub Actions configuration for Playwright
name: Playwright Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
- name: Install dependencies
run: npm ci
- name: Install Playwright browsers
run: npx playwright install --with-deps
- name: Run Playwright tests
run: npx playwright test
- uses: actions/upload-artifact@v3
if: always()
with:
name: playwright-report
path: playwright-report/
retention-days: 30
Selenium is compatible with virtually all CI/CD providers but offers less detailed guidance for integration. While it can be effectively integrated into any CI/CD pipeline, the implementation often requires more custom configuration:
# Example GitHub Actions configuration for Selenium
name: Selenium Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: actions/setup-java@v3
with:
distribution: 'temurin'
java-version: '17'
- name: Build with Maven
run: mvn -B package
- name: Run Selenium tests
run: mvn test
- uses: actions/upload-artifact@v3
if: always()
with:
name: test-reports
path: target/surefire-reports/
retention-days: 30
For teams prioritizing rapid CI/CD setup with minimal configuration, Playwright's comprehensive documentation and sample configurations provide a significant advantage.
11. Visual comparison testing
Visual comparison testing verifies that applications not only function correctly but also appear as expected to users.
Playwright includes built-in support for visual comparisons:
// Playwright visual testing
// Take a screenshot
await expect(page).toHaveScreenshot('homepage.png');
// Compare specific element
await expect(page.getByRole('navigation')).toHaveScreenshot('nav.png');
// With mask and style options
await expect(page).toHaveScreenshot('checkout.png', {
mask: [page.getByTestId('dynamic-content')],
style: 'body { animation: none !important; }',
});
These capabilities allow teams to detect unexpected visual changes without additional third-party tools. Playwright automatically generates reference screenshots during the first run and compares against them in subsequent runs. The framework includes pixel-by-pixel comparison with configurable threshold settings to handle minor rendering variations.
Selenium does not provide native visual testing capabilities. Implementing visual testing with Selenium requires integration with third-party tools like Applitools, Percy, or open-source libraries:
// Selenium + third-party visual testing
// Using an imaginary visual testing library
VisualTesting visualTesting = new VisualTesting(driver);
visualTesting.check("Homepage", driver.findElement(By.tagName("body")));
This requirement for external tools adds complexity to the testing stack and may increase costs. For applications where visual consistency is critical, Playwright's built-in visual testing capabilities offer a more streamlined solution.
12. Test runner support
Playwright includes a full-featured test runner called Playwright Test:
// Playwright Test example
import { test, expect } from '@playwright/test';
test.describe('Authentication', () => {
test('should allow login with valid credentials', async ({ page }) => {
await page.goto('/login');
await page.getByLabel('Username').fill('user@example.com');
await page.getByLabel('Password').fill('password123');
await page.getByRole('button', { name: 'Log in' }).click();
await expect(page.getByText('Welcome back')).toBeVisible();
});
test('should show error with invalid credentials', async ({ page }) => {
await page.goto('/login');
await page.getByLabel('Username').fill('wrong@example.com');
await page.getByLabel('Password').fill('wrongpassword');
await page.getByRole('button', { name: 'Log in' }).click();
await expect(page.getByText('Invalid credentials')).toBeVisible();
});
});
This built-in runner includes:
- Parallel test execution
- Test filtering and tagging
- Fixtures for setup/teardown
- Parameterized tests
- Comprehensive reporting
While Playwright can be used with other test runners like Jest and Mocha, its native test runner is optimized for web testing scenarios.
Selenium does not include a built-in test runner, requiring integration with framework-specific runners:
// Selenium with JUnit
@Test
public void testLogin() {
driver.get("/login");
driver.findElement(By.id("username")).sendKeys("user@example.com");
driver.findElement(By.id("password")).sendKeys("password123");
driver.findElement(By.id("login-button")).click();
assertTrue(driver.findElement(By.id("welcome-message")).isDisplayed());
}
While this approach offers flexibility to use any test framework, it requires additional setup and configuration. For teams looking to minimize setup time and leverage test runners optimized for web testing, Playwright's integrated solution offers clear advantages.
13. Record and playback support
Record and playback functionality accelerates test creation by allowing testers to generate test scripts through manual browser interactions.
Playwright provides a powerful codegen tool:
npx playwright codegen https://example.com
This launches a browser window where user interactions are automatically converted into executable test code in real-time. The tool supports:
- Multiple programming languages (JavaScript, Python, Java, .NET)
- Custom selectors through right-click options
- Editing generated assertions
- Directly copying code to clipboard
This approach enables rapid test prototyping while generating maintainable code that follows Playwright best practices.
Selenium offers record and playback through Selenium IDE, a browser extension:
// Example of Selenium IDE generated code
driver.get("https://example.com");
driver.findElement(By.id("username")).click();
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).click();
driver.findElement(By.id("password")).sendKeys("password");
driver.findElement(By.id("login")).click();
While functional, Selenium IDE is separated from the main Selenium WebDriver development experience and sometimes generates code that doesn't follow best practices for long-term maintainability.
For efficient test creation that balances rapid development with sustainable test code, Playwright's codegen offers advantages in generating high-quality, maintainable tests.
Comparison Table
Feature | Playwright | Selenium |
---|---|---|
Release date | 2020 | 2004 |
GitHub popularity | 61,000+ stars | Widely adopted |
Ease of installation | ✔️✔️ (Automated setup) | ✔️ (Manual setup required) |
Performance (avg time) | 4.513 seconds | 4.590 seconds |
Learning curve | ✔️✔️ (Intuitive API) | ✔️✔️ (Well-documented) |
Language support | JavaScript, Python, .NET, Java | C#, Python, Ruby, Kotlin, JavaScript, Java |
Debugging tools | ✔️✔️ (Inspector, trace viewer, VSCode integration) | ✔️ (Basic logging, IDE breakpoints) |
Browser support - Chromium | ✔️✔️ | ✔️✔️ |
Browser support - Firefox | ✔️✔️ | ✔️✔️ |
Browser support - Webkit | ✔️✔️ | ✔️✔️ |
Browser support - Edge | ✔️✔️ | ✔️✔️ |
Legacy browser support | ✖️ | ✔️✔️ (Including IE) |
Auto waiting | ✔️✔️ (Built-in) | ✖️ (Manual implementation) |
Retries | ✔️✔️ (Configurable) | ✖️ (Manual implementation) |
Multiple tabs/windows | ✔️✔️ | ✔️✔️ |
Test isolation | ✔️✔️ (BrowserContexts) | ✖️ (Manual implementation) |
Scalability | ✔️✔️ (Auto-parallel, sharding) | ✔️✔️ (Selenium Grid) |
CI/CD integration | ✔️✔️ (Detailed examples) | ✔️✔️ (Compatible, limited docs) |
Visual comparison testing | ✔️✔️ (Native support) | ✖️ (Third-party tools needed) |
Test runner | ✔️✔️ (Built-in) | ✖️ (Requires third-party) |
Record and playback | ✔️✔️ (Codegen) | ✔️ (Selenium IDE) |
Mobile testing | ✔️✔️ (Via emulation) | ✔️✔️ (Via Appium) |
Shadow DOM support | ✔️✔️ | ✔️ (Limited) |
iFrame support | ✔️✔️ | ✔️ (More complex setup) |
✖️ - No support ✔️ - Partial support ✔️✔️ - Full support
Final thoughts
Both Playwright and Selenium are powerful E2E testing tools with distinct advantages. Playwright excels in modern testing scenarios with its comprehensive built-in features, superior debugging capabilities, and seamless integration with contemporary development workflows. Its architecture, designed specifically for today's web applications, provides advantages in areas like auto-waiting, test isolation, and visual testing without requiring additional tools or custom implementations.
Selenium, with its long history and widespread adoption, offers unmatched language support and compatibility with legacy browsers. Its mature ecosystem includes extensive community resources, third-party integrations, and battle-tested solutions for enterprise-scale testing challenges.
For new projects prioritizing developer experience, test reliability, and built-in modern testing features, Playwright generally offers a more comprehensive out-of-the-box solution. For teams with existing Selenium expertise, projects requiring support for legacy browsers, or environments with specific language requirements not supported by Playwright, Selenium remains a robust and flexible choice.
Ultimately, the best tool depends on your specific testing requirements, team expertise, and application characteristics. Both frameworks continue to evolve, with Playwright rapidly adding features and Selenium maintaining its position as an industry standard through continuous improvement.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us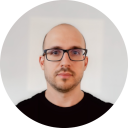
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github