Playwright vs Puppeteer: The Definitive Comparison
In the fast-paced world of web development, ensuring applications work flawlessly across browsers and devices is critical for user retention and business success.
End-to-end (E2E) testing plays a vital role in this process, allowing developers to verify that all components of an application function correctly together from a user's perspective.
This comprehensive guide examines two prominent browser automation and testing frameworks: Playwright and Puppeteer.
While these tools share common ancestry (Playwright was created by the original Puppeteer team) they have evolved to serve different testing needs in the modern development ecosystem.
Let's get started!
What is Playwright?
Playwright is a modern testing framework developed by Microsoft. Released in 2020, it quickly gained popularity with over 71,000 GitHub stars and 13 million weekly NPM downloads. This rapid adoption demonstrates the industry's recognition of Playwright's capabilities and reliability.
Playwright operates through the DevTools Protocol, which enables direct communication with browsers at a low level. This approach allows for more precise control over browser behavior and enables advanced features like network interception, geolocation mocking, and permission handling.
It supports all major browser engines (Chromium, WebKit, and Firefox) across Windows, Linux, and macOS, ensuring comprehensive coverage of user environments.
What is Puppeteer?
Puppeteer is a robust end-to-end testing framework introduced by Google in 2018. With over 3 million weekly downloads on NPM, it has established itself as a significant player in the browser automation landscape.
As a Node.js library, Puppeteer allows developers to use JavaScript to create detailed tests and effectively automate browser operations. It also uses the DevTools Protocol to control browsers, primarily focusing on Chromium-based browsers.
Puppeteer offers a wide array of high-level APIs for tasks like capturing screenshots, debugging performance, navigating SPAs, and managing automated form submissions and keyboard inputs. While initially designed for Chrome automation, its capabilities have expanded to include limited support for Firefox and other browsers.
Let's explore how these powerful tools compare across key evaluation criteria:
1. Ease of installation
Playwright provides an exceptionally streamlined installation experience. It requires only the relevant programming language environment (e.g., Node.js for JavaScript implementations).
A single command installs Playwright and automatically downloads all necessary
browsers. It also provides a sample test in the tests
directory and
automatically creates a Playwright configuration file.
This "batteries included" approach means you can start writing and running tests within minutes of installation.
npm init playwright@latest
After running this command, Playwright guides you through a setup wizard that helps configure your testing environment according to your preferences, including selecting browsers and test runners.
Puppeteer offers a straightforward installation process but with fewer automated setup features. Installing Puppeteer via npm automatically downloads a compatible version of Chromium:
npm install puppeteer
However, unlike Playwright, Puppeteer does not automatically set up the
directory structure or provide sample tests. You must manually configure your
project structure and create your first test files. For Firefox support, you
need to install the additional puppeteer-firefox
package.
While both tools offer relatively simple installation processes, Playwright's automated setup wizard and multi-browser installation provide a more comprehensive out-of-the-box experience, especially for teams looking to quickly implement cross-browser testing.
2. Performance
Performance is crucial in testing frameworks as faster execution times lead to shorter feedback loops during development. Based on carefully conducted benchmarks involving navigation to a local application and checking for specific elements, Playwright demonstrated superior performance with an average execution time of 4.513 seconds compared to Puppeteer's 4.784 seconds.
While the difference of 0.271 seconds might seem minimal in a single test, this performance gap becomes more significant when scaled to hundreds or thousands of tests, potentially saving substantial time in large test suites. Playwright's architecture, built specifically for modern browsers and optimizing the DevTools Protocol implementation, contributes to this performance edge.
Here's an example of how a test looks using Playwright:
const { test, expect } = require("@playwright/test");
test("has title", async ({ page }) => {
await page.goto("http://localhost:3000/");
// Expect the title to contain a substring.
await expect(page).toHaveTitle(/Book List/);
});
And the equivalent in Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('http://localhost:3000/');
const title = await page.title();
console.log('Title contains "Book List":', title.includes('Book List'));
await browser.close();
})();
The performance difference becomes particularly noticeable in CI/CD pipelines, where fast test execution is essential for continuous delivery workflows. While Puppeteer performs admirably, Playwright's slight speed advantage may benefit high-volume testing environments.
3. Learning curve and language support
The ease of learning and breadth of language support significantly impact adoption and team productivity.
Playwright features an intuitive API designed with modern development practices in mind. Its documentation is comprehensive, covering advanced capabilities like support for web components, shadow DOM, and iframes with clear examples.
Playwright's code completion and intelligent suggestions in IDEs like Visual Studio Code further flatten the learning curve. It supports multiple programming languages including JavaScript/TypeScript, Python, .NET, and Java, accommodating diverse development teams.
# Playwright Python example
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto("https://example.com")
print(page.title())
browser.close()
Puppeteer provides a straightforward API that shares many similarities with Playwright (which is not surprising as Playwright was created by the original Puppeteer team). This familiarity can ease the transition between the two tools. However, Puppeteer currently only supports JavaScript/TypeScript, limiting its applicability in polyglot development environments.
// Puppeteer JavaScript example
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
console.log(await page.title());
await browser.close();
})();
For teams working exclusively with JavaScript, both tools offer relatively gentle learning curves with well-documented APIs. However, for organizations using multiple programming languages, Playwright's multi-language support provides significant advantages in standardizing testing approaches across different projects and teams.
4. Debugging tools
Effective debugging capabilities significantly impact testing efficiency by reducing the time spent diagnosing and fixing issues.
Playwright excels with a comprehensive suite of debugging tools:
- VSCode Debugger Integration: Enables direct test step-through in the popular code editor.
- Playwright Inspector: A graphical interface that allows step-by-step test execution, live locator editing, and actionability log viewing.
- Trace Viewer: A sophisticated tool for examining recorded traces of test executions, including screenshots, DOM snapshots, and network requests.
- Browser Developer Tools: Running Playwright in debug mode exposes the browser's native developer tools.
- Verbose API Logs: Detailed logging of all API interactions when the DEBUG environment variable is set.
- Headed Mode with Slow-Motion: Tests can run visually with configurable execution speed for easier observation.
These tools work together to provide a multi-dimensional view of test execution, making it easier to identify issues ranging from timing problems to selector failures.
// Trace recording in Playwright
await page.goto('https://example.com');
await page.screenshot({ path: 'screenshot.png' });
await context.tracing.stop({ path: 'trace.zip' });
// This trace file can be opened in the Playwright Trace Viewer
Puppeteer offers more basic debugging capabilities:
- Headed Mode: Running browsers in non-headless mode to visually observe test execution.
- Slow-Motion: Slowing down operations to better observe interactions.
- Console Integration: Capturing and redirecting browser console output to Node.js.
- Screenshots and PDFs: Capturing visual state at specific points in test execution.
// Basic debugging in Puppeteer
const browser = await puppeteer.launch({
headless: false, // Run in headed mode for visual debugging
slowMo: 100 // Slow down operations by 100ms
});
// Capture console logs
page.on('console', msg => console.log('Browser console:', msg.text()));
// Take screenshots for debugging
await page.screenshot({ path: 'debug-screenshot.png' });
While Puppeteer's debugging tools are functional, they lack the integration and sophistication of Playwright's suite. For complex applications or intricate test scenarios, Playwright's comprehensive debugging toolkit can significantly reduce troubleshooting time and effort.
5. Browser support
Playwright offers exceptional cross-browser support, covering all major rendering engines:
- Chromium (including Chrome and Edge): Full support with advanced capabilities.
- Firefox: Complete support with consistent API across browsers.
- WebKit (Safari): Native support without requiring additional setup.
Additionally, Playwright provides robust mobile viewport emulation for testing responsive designs and mobile user experiences. It offers granular control over browser versions and updates, allowing tests to run against specific browser versions as needed. This flexibility is valuable for applications that must support particular browser environments.
Puppeteer was originally designed specifically for Chrome/Chromium automation:
- Chromium (including Chrome): Full support with advanced capabilities.
- Firefox: Experimental support with some limitations.
- WebKit (Safari): No native support.
- Edge: Limited support through Chromium-based Edge.
While Puppeteer has expanded to include experimental Firefox support, its capabilities remain most robust with Chromium-based browsers. For teams that need to test across multiple browser engines, particularly WebKit for Safari users, this limitation can be significant.
Both tools provide mobile viewport emulation for responsive testing, but Playwright's comprehensive cross-browser support makes it more suitable for applications that need to ensure consistent functionality across different browser engines.
6. Auto waiting
Auto-waiting mechanisms ensure that actions are performed only when elements are ready for interaction, significantly reducing flaky tests caused by timing issues.
Playwright implements sophisticated auto-waiting through its locator methods
like getByRole()
, page.getByLabel()
, and page.getByAltText()
. Before
performing actions, Playwright automatically:
- Checks element visibility.
- Verifies element stability (not moving).
- Confirms the element is enabled.
- Ensures the element is editable (for input-related actions).
If these conditions aren't met within a configurable timeout, Playwright aborts the action with a descriptive error message. This comprehensive approach eliminates most timing-related failures without requiring explicit waits in test code.
// Playwright automatically waits for the button to be visible, stable, and enabled
await page.getByRole('button', { name: 'Submit' }).click();
Puppeteer lacks robust built-in auto-waiting mechanisms. While it provides
methods like waitForSelector()
and waitForFunction()
, they require explicit
implementation in test code. This means developers must manually add waiting
logic throughout their tests:
// Puppeteer requires explicit waiting commands
await page.waitForSelector('#submit-button');
await page.click('#submit-button');
The experimental Puppeteer Locators API is beginning to address this limitation by providing some auto-waiting capabilities, but it's not yet as mature or comprehensive as Playwright's implementation.
This difference in auto-waiting capabilities is one of the most significant distinctions between the two frameworks. Playwright's automatic handling of element actionability significantly reduces the code needed to create stable tests and minimizes the expertise required to write timing-resilient test scripts.
7. Retries
Test retry capabilities help distinguish between genuine bugs and intermittent failures caused by environmental factors, network issues, or timing problems.
Playwright offers comprehensive retry functionality:
- Locator retries: Built into methods like
page.getByRole()
, which automatically retry finding elements - Global retry configuration: Set maximum retry attempts for all tests
- Test-specific overrides: Configure specific retry settings for individual tests or test groups
- Detailed reporting: Tests are categorized as "passed" (successful initially), "flaky" (fails initially but succeeds on retry), or "failed" (never passes)
This multi-layered approach allows teams to identify and address flaky tests while preventing them from blocking CI/CD pipelines.
// Playwright test retry configuration
// In playwright.config.js
module.exports = {
retries: 3, // Retry failed tests up to 3 times
};
// For a specific test
test('my flaky test', { retries: 5 }, async ({ page }) => {
// This test will retry up to 5 times
});
Puppeteer does not provide built-in retry mechanisms for tests. The experimental Locators API does include some retry logic at the element selection level, but comprehensive test retry capabilities require custom implementation:
// Custom retry logic needed with Puppeteer
const maxRetries = 3;
let retries = 0;
async function runTestWithRetry() {
try {
await runTest();
} catch (error) {
if (retries < maxRetries) {
retries++;
console.log(`Test failed, retrying (${retries}/${maxRetries})...`);
await runTestWithRetry();
} else {
throw new Error(`Test failed after ${maxRetries} retries: ${error.message}`);
}
}
}
Alternatively, teams can integrate Puppeteer with test runners like Jest or Mocha that support retry functionality, but this requires additional configuration and doesn't provide the same level of integration as Playwright's built-in solution.
Playwright's built-in retry capabilities significantly reduce the engineering effort required to manage test flakiness, particularly in large test suites running in CI/CD environments.
8. Multiple tabs
Support for multiple tabs or windows is essential for testing complex user journeys that span across different browser contexts.
Playwright provides elegant handling of multiple tabs within browser contexts:
// Playwright multiple tab handling
const context = await browser.newContext();
// Create two pages within the same context
const pageOne = await context.newPage();
const pageTwo = await context.newPage();
await pageOne.goto('https://example.com');
await pageTwo.goto('https://another-example.com');
// Interact with both pages
await pageOne.getByRole('button', { name: 'Login' }).click();
await pageTwo.getByRole('link', { name: 'Register' }).click();
// Retrieve all pages of a browser context
const allPages = context.pages();
This approach makes it straightforward to create and manage multiple tabs that share the same session state, cookies, and local storage—ideal for testing features like "open in new tab" functionality.
Puppeteer also supports working with multiple tabs simultaneously:
// Puppeteer multiple tab handling
const browser = await puppeteer.launch();
// Create two pages
const pageOne = await browser.newPage();
const pageTwo = await browser.newPage();
await pageOne.goto('https://example.com');
await pageTwo.goto('https://another-example.com');
// Interact with both pages
await pageOne.click('button[name="Login"]');
await pageTwo.click('a[name="Register"]');
// Retrieve all pages
const allPages = await browser.pages();
Both frameworks effectively support multiple tabs, with similar APIs for creating and managing pages. The key difference lies in Playwright's context-based approach, which provides better isolation between different browser states and more closely mimics real user behavior across multiple tabs.
9. Test isolation
Test isolation ensures that each test runs in a clean environment, preventing tests from affecting each other and making test failures more reproducible.
Playwright excels in test isolation through its BrowserContext concept:
// Playwright test isolation
test('first test', async ({ browser }) => {
// This creates a fresh context for this test only
const context = await browser.newContext();
const page = await context.newPage();
// Any cookies, localStorage, etc. are isolated to this context
await page.goto('https://example.com');
await context.close();
});
test('second test', async ({ browser }) => {
// This test gets its own fresh context
const context = await browser.newContext();
const page = await context.newPage();
// No shared state with the previous test
await page.goto('https://example.com');
await context.close();
});
These contexts function like separate user profiles or incognito windows, but they're much lighter and faster to create. Each test gets a pristine environment with fresh cookies, local storage, session storage, and cache, preventing cross-test contamination.
Puppeteer also supports browser contexts for test isolation:
// Puppeteer test isolation
async function runIsolatedTest1() {
const browser = await puppeteer.launch();
// Create a new browser context for isolation
const context = await browser.createIncognitoBrowserContext();
const page = await context.newPage();
// State is isolated to this context
await page.goto('https://example.com');
await context.close();
await browser.close();
}
async function runIsolatedTest2() {
const browser = await puppeteer.launch();
// Create a new browser context for isolation
const context = await browser.createIncognitoBrowserContext();
const page = await context.newPage();
// No shared state with the previous test
await page.goto('https://example.com');
await context.close();
await browser.close();
}
While both frameworks support the concept of isolated browser contexts, Playwright's integration of context creation with its test runner makes isolation more automatic and less prone to implementation errors. In Puppeteer, developers must remember to explicitly create and manage these contexts, which can lead to inconsistent isolation practices across a test suite.
10. Scalability
Scalability determines how effectively a testing framework can handle large test suites and distribute test execution across computing resources.
Playwright offers excellent scalability features:
- Automatic parallelization: Runs test files in parallel across available CPU cores by spawning worker processes.
- Fine-grained control: Allows parallelizing tests within a single file if needed.
- Test sharding: Supports distributing tests across multiple machines to reduce execution time.
- Worker isolation: Each test worker runs in isolation to prevent interference.
// Playwright parallelization configuration
// In playwright.config.js
module.exports = {
workers: 8, // Run tests in 8 parallel workers
// Alternatively, use a percentage of CPU cores
// workers: '50%',
};
For distributed execution across multiple machines:
# On machine 1
npx playwright test --shard=1/3
# On machine 2
npx playwright test --shard=2/3
# On machine 3
npx playwright test --shard=3/3
Puppeteer lacks built-in support for test parallelization or sharding. While it's possible to implement custom solutions using worker threads or multiple processes, these approaches require significant additional development:
// Custom parallelization with Puppeteer requires manual implementation
const { Worker, isMainThread, workerData } = require('worker_threads');
if (isMainThread) {
// Distribute tests across worker threads
const numWorkers = 4;
for (let i = 0; i < numWorkers; i++) {
const worker = new Worker(__filename, {
workerData: {
workerId: i,
totalWorkers: numWorkers
}
});
}
} else {
// In worker thread, run a subset of tests
const { workerId, totalWorkers } = workerData;
// Run tests assigned to this worker
}
Alternatively, Puppeteer can be integrated with test runners like Jest that support parallelization, but this still doesn't provide the test sharding capabilities needed for distributed execution across multiple machines.
For large-scale testing, Playwright's built-in parallelization and sharding capabilities provide significant advantages in terms of execution speed and resource utilization.
11. CI/CD integration
Playwright provides exceptional CI/CD support with detailed documentation and sample configurations for major providers:
- GitHub Actions
- Azure Pipelines
- CircleCI
- Jenkins
- GitLab CI
These configurations include features like:
- Parallel test execution
- Report generation and merging
- Artifact storage for screenshots and videos
- Cache optimization for faster runs
# Example GitHub Actions configuration for Playwright
name: Playwright Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
- name: Install dependencies
run: npm ci
- name: Install Playwright browsers
run: npx playwright install --with-deps
- name: Run Playwright tests
run: npx playwright test
- uses: actions/upload-artifact@v3
if: always()
with:
name: playwright-report
path: playwright-report/
retention-days: 30
Puppeteer can be integrated with various CI/CD providers, but offers less detailed guidance for integration. While examples exist for some providers like GitHub Actions, they generally lack the comprehensiveness of Playwright's documentation:
# Example GitHub Actions configuration for Puppeteer
name: Puppeteer Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
- name: Install dependencies
run: npm ci
- name: Run Puppeteer tests
run: npm test
- uses: actions/upload-artifact@v3
if: failure()
with:
name: screenshots
path: screenshots/
retention-days: 7
While both frameworks can be effectively integrated into CI/CD pipelines, Playwright's comprehensive documentation and sample configurations make it easier to implement robust automated testing workflows, especially for teams with limited DevOps expertise.
12. Visual comparison testing
Visual comparison testing verifies that applications not only function correctly but also appear as expected to users.
Playwright includes built-in support for visual comparisons:
// Playwright visual testing
// Take a screenshot
await expect(page).toHaveScreenshot('homepage.png');
// Compare specific element
await expect(page.getByRole('navigation')).toHaveScreenshot('nav.png');
// With mask and style options
await expect(page).toHaveScreenshot('checkout.png', {
mask: [page.getByTestId('dynamic-content')],
style: 'body { animation: none !important; }',
});
These capabilities allow teams to detect unexpected visual changes without additional third-party tools. Playwright automatically generates reference screenshots during the first run and compares against them in subsequent runs. The framework includes pixel-by-pixel comparison with configurable threshold settings to handle minor rendering variations.
Puppeteer does not provide built-in visual comparison functionality. Implementing visual testing with Puppeteer typically requires integration with third-party libraries:
// Puppeteer with jest-image-snapshot for visual testing
const { toMatchImageSnapshot } = require('jest-image-snapshot');
expect.extend({ toMatchImageSnapshot });
// In your test
const screenshot = await page.screenshot();
expect(screenshot).toMatchImageSnapshot();
This approach works but requires additional setup and maintenance of the visual testing infrastructure. For teams that prioritize visual regression testing as part of their quality assurance process, Playwright's built-in capabilities offer a more streamlined solution.
13. Test runner support
Integrated test runners simplify the process of organizing, executing, and reporting test results.
Playwright includes a full-featured test runner called Playwright Test:
// Playwright Test example
import { test, expect } from '@playwright/test';
test.describe('Authentication', () => {
test('should allow login with valid credentials', async ({ page }) => {
await page.goto('/login');
await page.getByLabel('Username').fill('user@example.com');
await page.getByLabel('Password').fill('password123');
await page.getByRole('button', { name: 'Log in' }).click();
await expect(page.getByText('Welcome back')).toBeVisible();
});
test('should show error with invalid credentials', async ({ page }) => {
await page.goto('/login');
await page.getByLabel('Username').fill('wrong@example.com');
await page.getByLabel('Password').fill('wrongpassword');
await page.getByRole('button', { name: 'Log in' }).click();
await expect(page.getByText('Invalid credentials')).toBeVisible();
});
});
This built-in runner includes:
- Parallel test execution
- Test filtering and tagging
- Fixtures for setup/teardown
- Parameterized tests
- Comprehensive reporting
While Playwright can be used with other test runners like Jest and Mocha, its native test runner is optimized for web testing scenarios.
Puppeteer does not include a built-in test runner. Tests must be integrated with third-party testing frameworks like Jest, Mocha, or Jasmine:
// Puppeteer with Jest
const puppeteer = require('puppeteer');
describe('Authentication', () => {
let browser;
let page;
beforeAll(async () => {
browser = await puppeteer.launch();
});
beforeEach(async () => {
page = await browser.newPage();
await page.goto('http://localhost:3000/login');
});
afterAll(async () => {
await browser.close();
});
test('should allow login with valid credentials', async () => {
await page.type('#username', 'user@example.com');
await page.type('#password', 'password123');
await page.click('#login-button');
await page.waitForSelector('#welcome-message');
const text = await page.$eval('#welcome-message', el => el.textContent);
expect(text).toContain('Welcome back');
});
});
While this approach offers flexibility to use any test framework, it requires additional setup and configuration. For teams looking to minimize setup time and leverage test runners optimized for web testing, Playwright's integrated solution offers clear advantages.
14. Record and playback support
Record and playback functionality accelerates test creation by allowing testers to generate test scripts through manual browser interactions.
Playwright provides a powerful codegen tool:
npx playwright codegen https://example.com
This launches a browser window where user interactions are automatically converted into executable test code in real-time. The tool supports:
- Multiple programming languages (JavaScript, Python, Java, .NET)
- Custom selectors through right-click options
- Editing generated assertions
- Directly copying code to clipboard
This approach enables rapid test prototyping while generating maintainable code that follows Playwright best practices.
Puppeteer offers more limited record and playback capabilities. While developers can use Chrome DevTools' recorder to generate Puppeteer-compatible code, this approach is less integrated and may require manual adjustment of the generated scripts:
# Puppeteer lacks a dedicated codegen tool like Playwright's
# Chrome DevTools can be used as a workaround
For efficient test creation that balances rapid development with sustainable test code, Playwright's codegen offers advantages in generating high-quality, maintainable tests across multiple programming languages.
Comparison Table
Feature | Playwright | Puppeteer |
---|---|---|
Release date | 2020 | 2018 |
GitHub popularity | 71,000+ stars | 3+ million weekly NPM downloads |
Ease of installation | ✔️✔️ (Automated setup) | ✔️ (Basic setup) |
Performance (avg time) | 4.513 seconds | 4.784 seconds |
Learning curve | ✔️✔️ (Intuitive API) | ✔️✔️ (Familiar API) |
Language support | JavaScript, Python, .NET, Java | JavaScript only |
Debugging tools | ✔️✔️ (Inspector, trace viewer, VSCode integration) | ✔️ (Basic tools) |
Browser support - Chromium | ✔️✔️ | ✔️✔️ |
Browser support - Firefox | ✔️✔️ | ✔️ (Experimental) |
Browser support - Webkit | ✔️✔️ | ✖️ |
Auto waiting | ✔️✔️ (Built-in) | ✖️ (Manual implementation) |
Retries | ✔️✔️ (Configurable) | ✖️ (Manual implementation) |
Multiple tabs/windows | ✔️✔️ | ✔️✔️ |
Test isolation | ✔️✔️ (BrowserContexts) | ✔️✔️ (IncognitoBrowserContext) |
Scalability | ✔️✔️ (Auto-parallel, sharding) | ✖️ (Manual implementation) |
CI/CD integration | ✔️✔️ (Detailed examples) | ✔️ (Limited documentation) |
Visual comparison testing | ✔️✔️ (Native support) | ✖️ (Third-party tools needed) |
Test runner | ✔️✔️ (Built-in) | ✖️ (Requires third-party) |
Record and playback | ✔️✔️ (Codegen) | ✔️ (Chrome DevTools workaround) |
Mobile testing | ✔️✔️ (Via emulation) | ✔️ (Limited device emulation) |
Shadow DOM support | ✔️✔️ | ✔️ (Basic support) |
iFrame support | ✔️✔️ | ✔️ (More complex setup) |
✖️ - No support ✔️ - Partial support ✔️✔️ - Full support
Final thoughts
Both Playwright and Puppeteer are powerful E2E testing tools with distinct advantages. Playwright, though newer, offers a more comprehensive feature set that addresses many of the limitations in Puppeteer. Its multi-browser support, built-in test runner, sophisticated auto-waiting mechanisms, and parallelization capabilities make it well-suited for complex, large-scale testing requirements.
Puppeteer, as the predecessor to Playwright (developed by many of the same team members), provides solid functionality for Chrome-based browser automation and remains a valid choice for simpler testing needs or teams deeply invested in existing Puppeteer implementations. Its tight integration with Chrome makes it particularly effective for Chrome-specific testing or performance profiling.
Interestingly, the relationship between these tools is not purely competitive. Playwright can be seen as the evolution of Puppeteer, incorporating lessons learned from years of browser automation experience. This is why they share many API similarities, making migration from Puppeteer to Playwright relatively straightforward for teams looking to leverage the additional capabilities.
For new projects, particularly those requiring cross-browser testing or looking to leverage advanced test automation features, Playwright generally offers a more comprehensive solution. For teams with existing Puppeteer expertise working primarily with Chrome, continuing with Puppeteer may be practical for simpler use cases, with the option to migrate to Playwright as testing needs grow more complex.
Both frameworks continue to evolve, with Playwright rapidly adding features and Puppeteer maintaining its position as a reliable tool for Chrome automation through continuous improvement.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us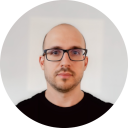
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github