What Does the “yield” Keyword Do in Python?
To better understand what yield
does, you need first to understand what generator
and iterable
are.
What is iterable
When you use a list or list-like structure, you can read the values from the list one by one. This is called iteration.
list_of_numbers = [1,10,100,1000]
# iterating over the list
for i in list_of_numbers:
print(i)
This is why in many languages, the iterating variable is usually named i
, but it can be named however you like. In short, basically, anything that can be used in for _ in _
is iterable.
What is generator
Generators are special kinds of iterables. They don’t store the values in memory, but rather generate the value on the fly. This means you can only iterate over them once.
generator = (x + 1 for x in range(4))
for i in generator:
print(i)
What is yield
Finally the yield
keyword. yield
is used like return but instead of returning a single value it returns a generator. This is very useful when your function will return a huge set of values that you don’t want to store in memory.
def create_generator(n):
for i in range(n):
yield n + 1
generator = create_generator(4)
for i in generator:
print(i)
In the example above, when calling the function create_generator
, the function doesn’t return, instead, it creates a generator object.
-
How To Log All Requests From The Python Request Library?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to log all requests from the python request li...
Questions -
How To Write Logs To A File With Python?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to write logs to a file in Python: Using Basic...
Questions -
Task scheduling in Python
Learn how to create and monitor Python scheduled tasks in a production environment
Guides -
How to Log to Stdout with Python?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to log to Stdout with Python: Using Basic Conf...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us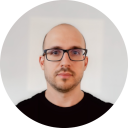
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github