Replacements for switch statement in Python?
In Python, the if-elif-else
statement can be used as a replacement for a switch statement. Additionally, the dict.get()
method or a dictionary of functions can also be used to achieve a similar effect.
Here are a few examples of using the if-elif-else
statement as a replacement for a switch statement in Python:
def switch_example(case):
if case == 'a':
return 'Case A'
elif case == 'b':
return 'Case B'
elif case == 'c':
return 'Case C'
else:
return 'Other Case'
print(switch_example('a')) # 'Case A'
print(switch_example('b')) # 'Case B'
print(switch_example('c')) # 'Case C'
print(switch_example('d')) # 'Other Case'
Here is an example of using the dict.get()
method as a replacement for a switch statement in Python:
def switch_example(case):
options = {
'a': 'Case A',
'b': 'Case B',
'c': 'Case C',
}
return options.get(case, 'Other Case')
print(switch_example('a')) # 'Case A'
print(switch_example('b')) # 'Case B'
print(switch_example('c')) # 'Case C'
print(switch_example('d')) # 'Other Case'
And here is an example of using a dictionary of functions as a replacement for a switch statement in Python:
def case_a():
return 'Case A'
def case_b():
return 'Case B'
def case_c():
return 'Case C'
def other_case():
return 'Other Case'
def switch_example(case):
options = {
'a': case_a,
'b': case_b,
'c': case_c,
}
return options.get(case, other_case)()
print(switch_example('a')) # 'Case A'
print(switch_example('b')) # 'Case B'
print(switch_example('c')) # 'Case C'
print(switch_example('d')) # 'Other Case'
All of the above examples show different ways of achieving similar functionality as switch statements in python.
-
Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8. Here is an ex...
Questions -
Extracting extension from filename in Python
You can use the os.path.splitext() function from the os.path module in Python to extract the file extension from a filename. Here's an example: import os filename = "example.txt" extension = os.pat...
Questions -
How do I get the last element of a list in Python?
To get the last element of a list in Python, you can use the negative indexing feature of the list data type. For example: mylist = [1, 2, 3, 4, 5] lastelement = mylist[-1] # lastelement will be 5...
Questions -
How to limit floats to X decimal points in Python?
There are a few different ways to limit the number of decimal places for a float in Python. Here are three options: Use the round() function: x = 3.14159265 rounded = round(x, 2) # 3.14 Use string...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us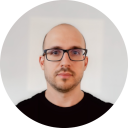
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github