Using node.js, How Do I Read a Json File into (Server) Memory?
In Node.js, you can read a JSON file into memory using the built-in fs
(File System) module to read the file content and then use JSON.parse
to parse the JSON data. Here's a simple example:
const fs = require('fs');
// Specify the path to your JSON file
const filePath = 'path/to/your/file.json';
// Read the JSON file
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error('Error reading JSON file:', err);
return;
}
// Parse the JSON data
try {
const jsonData = JSON.parse(data);
// Now you have the JSON data in memory
console.log('JSON Data:', jsonData);
} catch (parseError) {
console.error('Error parsing JSON:', parseError);
}
});
Explanation:
fs.readFile
: Reads the content of the file specified byfilePath
.'utf8'
: Specifies the file encoding. This is important when reading text files.- The callback function is called once the file is read. If there's an error, it's logged. If not, the data is passed to
JSON.parse
. JSON.parse
: Parses the JSON data into a JavaScript object.
Make sure to replace 'path/to/your/file.json'
with the actual path to your JSON file.
Keep in mind that reading files is an asynchronous operation in Node.js, and the callback function is used to handle the results. If you're working with modern JavaScript, you can also use util.promisify
or the fs.promises
API for a more synchronous-like code structure.
-
Best Node.js Application Monitoring Tools in 2023
Node.js Applications Performance Management and Monitoring tools enable code-level observability, faster recovery, troubleshooting, and easier maintenance of Node.js applications.
Comparisons -
How to read environment variables in Node.js
In Node.js, you can read environmental variables using the process.env object. This object provides access to the user environment, including environment variables. Here's a simple example of how y...
Questions -
How to get `GET` variables in Express.js on Node.js?
In Express.js, you can access GET (query string) variables using the req.query object. The req.query object contains key-value pairs of the query string parameters in a GET request. Here's an examp...
Questions -
How is an HTTP POST request made in node.js?
In Node.js, you can make an HTTP POST request using the http or https modules that come with Node.js, or you can use a more user-friendly library like axios or node-fetch. Here, I'll provide exampl...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us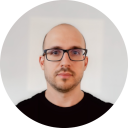
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github