How To Set Up And Secure Prometheus Metrics Endpoints
Exposing Prometheus metrics is essential for monitoring, but securing these endpoints is crucial to prevent unauthorized access and protect sensitive data. Here’s how you can set up and secure Prometheus metrics endpoints effectively.
Setting Up Metrics Endpoints
To expose metrics, integrate a Prometheus client library into your application. For example, in Python with Flask, you can install the prometheus-client
library, define a /metrics
endpoint, and increment counters for tracking events.
from flask import Flask
from prometheus_client import Counter, generate_latest
app = Flask(__name__)
REQUEST_COUNT = Counter('http_requests_total', 'Total HTTP requests', ['method', 'endpoint'])
@app.route('/metrics')
def metrics():
return generate_latest(), 200, {'Content-Type': 'text/plain; charset=utf-8'}
@app.route('/')
def home():
REQUEST_COUNT.labels(method='GET', endpoint='/').inc()
return "Hello, World!"
if __name__ == "__main__":
app.run(host='0.0.0.0', port=5000)
In prometheus.yml
, configure Prometheus to scrape the endpoint:
scrape_configs:
- job_name: 'my-app'
static_configs:
- targets: ['<app-ip>:5000']
Securing Metrics Endpoints
Basic Authentication
Protect the /metrics
endpoint with basic authentication using a reverse proxy like NGINX. First, create a password file with htpasswd
and then configure NGINX:
server {
listen 5000;
location /metrics {
proxy_pass http://localhost:5000/metrics;
auth_basic "Restricted Access";
auth_basic_user_file /etc/nginx/.htpasswd;
}
}
Update Prometheus to include credentials in the scrape configuration:
basic_auth:
username: 'prometheus_user'
password: '<password>'
IP Whitelisting
Restrict access to trusted IP ranges using a reverse proxy:
location /metrics {
allow 192.168.1.0/24;
deny all;
proxy_pass http://localhost:5000/metrics;
}
HTTPS and TLS
Use HTTPS to encrypt communication by generating SSL certificates with tools like OpenSSL. Modify the Flask app to use HTTPS:
app.run(host='0.0.0.0', port=5000, ssl_context=('cert.pem', 'key.pem'))
Update Prometheus to scrape metrics over HTTPS:
scheme: https
tls_config:
ca_file: /path/to/ca.crt
Token-Based Authentication
For more advanced security, use token-based authentication. Configure a reverse proxy to validate tokens and pass them in Prometheus:
authorization:
credentials: '<token>'
Kubernetes Security
In Kubernetes, secure metrics with RBAC and ServiceMonitors. Use NetworkPolicies to restrict access to the Prometheus pod.
Best Practices
- Always use HTTPS to secure data in transit.
- Implement authentication (basic or token-based) to restrict access.
- Avoid exposing metrics endpoints to the public internet; restrict to trusted networks.
- Regularly review and rotate credentials or tokens.
- Use Kubernetes-specific security features, such as RBAC and NetworkPolicies, when applicable.
Securing Prometheus metrics endpoints ensures reliable monitoring while protecting sensitive data from unauthorized access.
-
How to Add Custom HTTP Headers in Prometheus
Here is the content with only the indentation fixed: Adding custom HTTP headers in Prometheus is useful when interacting with a secured remote endpoint, such as when scraping metrics from services ...
Questions -
What is the Difference Between a Gauge and a Counter?
Gauges and counters are two core metric types in Prometheus. They serve different purposes and are used to track different kinds of data. 1. Counter A counter is a metric that only increases over t...
Questions -
How to Monitor REST APIs with Prometheus
Prometheus is an effective tool for monitoring REST APIs by collecting and analyzing metrics. By integrating Prometheus with your application or external monitoring setup, you can track key perform...
Questions -
What is a Prometheus target?
In Prometheus, a target is an endpoint or service that Prometheus monitors by scraping metrics. These metrics are exposed in a Prometheus-compatible format, typically over an HTTP or HTTPS endpoint...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us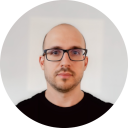
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github