How to Read a File Line-by-Line into a List?
You can read a file line-by-line into a list in Python using a loop to iterate over each line in the file and appending each line to a list. Here's how you can do it:
# Open the file for reading
with open('filename.txt', 'r') as file:
# Read lines from the file and store them in a list
lines = file.readlines()
# Print the list of lines
print(lines)
In this code:
'filename.txt'
is the name of the file you want to read. Replace it with the actual filename.'r'
specifies that the file should be opened in read mode.file.readlines()
reads all lines from the file and returns them as a list of strings, where each string represents one line of the file.- The list of lines is stored in the variable
lines
.
Now, the variable lines
contains a list where each element corresponds to a line from the file.
If you want to remove the newline characters ('\\n'
) from each line, you can use the rstrip()
method within a list comprehension like this:
# Open the file for reading
with open('filename.txt', 'r') as file:
# Read lines from the file, remove trailing newline characters, and store them in a list
lines = [line.rstrip('\\n') for line in file]
# Print the list of lines
print(lines)
This will give you a list where each line is stripped of any trailing newline characters.
-
How do I append to a file in Python?
To append to a file in Python, you can use the "a" mode in the open() function. This will open the file in append mode, which means that you can write new data at the end of the file. Here is an ex...
Questions -
How to Log to Stdout with Python?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to log to Stdout with Python: Using Basic Conf...
Questions -
How do I profile a Python script?
By default, Python contains a profiler called cProfile. It not only gives the total running time, but also times each function separately, and tells you how many times each function was called, mak...
Questions -
How to run Cron jobs in Python?
To run Cron jobs in Python, you can create a Python script with the code you want to run and schedule it to run using Cron. Here are the steps to create and schedule a Python script: 🔠Want to get ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us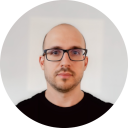
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github