How do I count the occurrences of a list item in Python?
You can use the count()
method of a list to count the number of occurrences of an item in the list.
For example:
my_list = ['a', 'b', 'a', 'c', 'a']
print( my_list.count('a') ) # 3
This will return 3, because the element 'a' occurs 3 times in the list.
You can also use the collections
module's Counter
class to count the occurrences of items in a list.
For example:
from collections import Counter
my_list = ['a', 'b', 'a', 'c', 'a']
count = Counter(my_list)
print( count['a'] ) # 3
This will return a Counter
object with the count of each element in the list. You can then access the count of a specific element using the element as the key.
Note that the count()
method only works on lists, while the Counter
class can be used to count the occurrences of items in any iterable.
-
Finding the index of an item in a Python list?
To find an index of the first occurrence of an element in a given list, you can use index method of List class with the element passed as an argument. The syntax is the following: my_list = [1,2,0,...
Questions -
How do I check if a list is empty in Python?
You can check if a list is empty by using the len() function to check the length of the list. If the length of the list is 0, then it is empty. Here's an example: my_list = [] if len(my_list) == 0:...
Questions -
How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the + operator or the extend() method. Here is an example using the + operator: list1 = [1, 2, 3] list2 = [4, 5, 6] list3 = list1 + list2 print(list...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us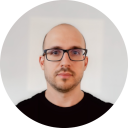
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github