Using async/await with a forEach loop?
In Node.js, the forEach
loop is not compatible with the async/await syntax as it does not wait for promises. But fear not, as there are two alternatives:
The for(… of …)
loop
If you would like to handle asynchronous operations in order one by one (that means sequentially) you can use the following syntax utilizing the for(const item in list)
syntax:
async function processInLoopAsync(list){
for (const item in list){
const result = await operationAsync(item);
console.log(result);
}
}
In this example, the asynchronous operation is
represented by an
async function operationAsync
. This asynchronous operation is being processed in a loop and each time we wait until the asynchronous operation is finished before we move to the next one. This will result in slower execution in comparison with parallel execution shown below, but it finds its use in cases where the next (async) operation might depend on the result of the previous one.
The Promise.all
with map
In this example, we will handle the asynchronous operation not one by one (sequentially) but we will process parallel - them all at once (sort of).
async function processParallelAsync(list) {
await Promise.all(list.map(async (item) => {
const result = await operationAsync(item);
console.log(result);
}));
}
In this example, the execution will not be stopped to wait for each promise to resolve, but rather wait for all of them to resolve at once. This is useful when we need to do multiple asynchronous operations that are independent of each other.
Choose whatever works best for you. If you have multiple independent async jobs that can be run parallel - choose Promise.all
. If you have multiple jobs where each one needs the previous one to finish - choose for
loop.
-
Logging in Nodejs
Learn how to start logging with Node.js and go from basics to best practices in no time.
Guides -
Node.js Clustering
Due to Node.js's architecture, deploying a Node.js application on a machine with multiple CPUs typically runs as a single instance on a single CPU, responsible for handling all incoming requests. T...
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us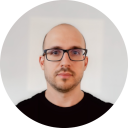
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github