How Do I Test a Single File Using Jest?
To test a single file using Jest, you can follow these steps:
Install Jest
If you haven't installed Jest yet, you can install it using npm:
npm install --save-dev jest
Create a Jest Configuration (Optional)
You can create a Jest configuration file (e.g., jest.config.js
) to specify your test file patterns, test environment, and other configurations. If you don't create a configuration file, Jest will use its defaults.
Example jest.config.js
:
module.exports = {
testEnvironment: 'node', // Use 'node' for Node.js environment
testMatch: ['<rootDir>/path/to/testfile.test.js'], // Adjust the path to your test file
};
Write Your Test File
Create your test file. Jest identifies test files based on their filename. For example, if your module is in a file named math.js
, the corresponding test file should be named math.test.js
or math.spec.js
.
// math.test.js
const { add, subtract } = require('./math');
test('add function', () => {
expect(add(2, 3)).toBe(5);
});
test('subtract function', () => {
expect(subtract(5, 2)).toBe(3);
});
Run Jest
Run Jest using the following command in your terminal:
npx jest
Jest will run the tests and report the results. If you want to run a specific test file, you can provide the path to that file as an argument:
npx jest path/to/your/testfile.test.js
Jest will execute the specified test file and provide the test results.
That's it! Jest will automatically detect and run your test file. The expect
function is used for assertions in Jest, and it provides various matchers for different types of assertions. Adjust the test file and configuration according to your project's needs.
-
Comparing Node.js Testing Libraries
This guide presents a comparison of top 9 testing libraries for Node.js to help you decide which one to use in your next project
Guides -
How Do I Test a Single File Using Node Built-in Test Runner?
Node.js itself does not come with a built-in test runner. However, you can use the built-in assert module for simple assertion-based tests. If you want a more feature-rich test runner, you might co...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us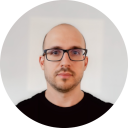
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github