How to Store node.js Deployment Settings/Configuration Files?
Storing deployment settings or configuration files for a Node.js application can be approached in several ways. The choice depends on your specific needs, the environment, and the level of security you require. Here are some common methods:
Environment Variables
Store configuration values as environment variables. This is a common practice for sensitive information like API keys or database credentials.
Use a package like dotenv
to load environment variables from a file during development.
const dotenv = require('dotenv');
dotenv.config(); // Load environment variables from a .env file during development
const apiKey = process.env.API_KEY;
Configuration Files
Use JSON or YAML files to store configuration settings. Load these files using a configuration module.
const config = require('./config.json');
const apiKey = config.apiKey;
Command-Line Arguments
Pass configuration values as command-line arguments when starting the Node.js application.
node app.js --apiKey=your-api-key
const args = require('yargs').argv;
const apiKey = args.apiKey;
Database or Key-Value Stores
Store configuration settings in a database or key-value store. Retrieve them when needed.
Examples: MongoDB, Redis, etcd.
Configuration Modules:
Create a dedicated module to manage and export configuration settings.
// config.js
module.exports = {
apiKey: 'your-api-key',
// other configuration settings...
};
// app.js
const config = require('./config');
const apiKey = config.apiKey;
Secret Management Tools
Use dedicated secret management tools like AWS Secrets Manager, HashiCorp Vault, or Azure Key Vault for secure storage of sensitive information.
Choose the method that best fits your application's requirements. It's common to use a combination of these approaches depending on the sensitivity and nature of the configuration data. Always be cautious with sensitive information and follow best practices for securing credentials and secrets.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us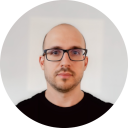
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github