How to Prettyprint a Json File in Python?
You can pretty print a JSON file in Python using the json
module's loads()
function to parse the JSON data, and then the json.dumps()
function with the indent
parameter to output it in a readable format. Here's how you can do it:
import json
# Load JSON data from a file
with open('file.json', 'r') as file:
json_data = json.load(file)
# Pretty print the JSON data
pretty_json = json.dumps(json_data, indent=4)
print(pretty_json)
In this code:
- Replace
'file.json'
with the path to your JSON file. json.load(file)
reads the JSON data from the file and parses it into a Python object (usually a dictionary or a list of dictionaries).json.dumps(json_data, indent=4)
converts the parsed JSON data back into a formatted JSON string with an indentation of 4 spaces, making it more readable.- The resulting formatted JSON string is stored in the variable
pretty_json
, which you can then print or manipulate further as needed.
This will output the JSON data in a nicely formatted, human-readable way.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us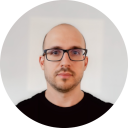
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github