How Can I Print a Circular Structure in a Json-like Format?
If you have an object with circular references in Node.js and you want to print it in a JSON-like format, you can use the util.inspect
method from the built-in util
module. The util.inspect
method provides options to handle circular references and format the output.
Here's an example:
const util = require('util');
const circularObject = {
prop1: 'value1',
prop2: {
prop3: 'value3',
},
};
// Create a circular reference
circularObject.circularRef = circularObject;
// Use util.inspect to print the object with circular references
const formattedOutput = util.inspect(circularObject, { depth: null });
console.log(formattedOutput);
In this example:
- The
util.inspect
method is used to print the object with circular references. - The
depth: null
option allows inspecting objects with circular references by setting the depth tonull
(unlimited depth). - The result is a formatted string that represents the object with circular references in a JSON-like format.
Adjust the options of util.inspect
according to your needs. The util.inspect
method is powerful and provides various options for formatting and customizing the output. Refer to the official documentation for more details on available options.
-
How to change node.js's console font color?
In Node.js, you can change the console font color by using ANSI escape codes. ANSI escape codes are sequences of characters that control text formatting, including colors. Here's a simple example o...
Questions -
Is there a map function for objects in Node.js?
In JavaScript, the map function is typically used with arrays to transform each element of the array based on a provided callback function. If you want to achieve a similar result with objects, you...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us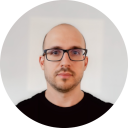
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github