How to parse nginx logs
Parsing Nginx logs is a common task for administrators and developers who want to analyze web server activity, monitor traffic, and troubleshoot issues. Nginx logs can provide valuable insights into server performance and user behavior. In this tutorial, we'll explore various methods to parse Nginx logs, including using command-line tools, log analyzers, and programming languages.
Prerequisites
Before we begin, make sure you have the following prerequisites:
- Nginx installed and configured.
- Access to the Nginx log files, typically located in
/var/log/nginx/
or a custom location.
Using Command-Line Tools
grep
and awk
You can use basic command-line tools like grep
and awk
to extract information from Nginx log files. Here's an example of how to count the number of requests for a specific URL:
grep "/your-url" /var/log/nginx/access.log | wc -l
To extract and display specific fields, you can use awk
:
awk '{print $1, $4, $7}' /var/log/nginx/access.log
sed
You can also use sed
to manipulate log data. For example, to extract IP addresses from the logs:
sed -nE 's/([^ ]+).+ - .+ \\[.+]\\s"GET \\/your-url.+/\\1/p' /var/log/nginx/access.log
Log Analyzers
Several log analyzers can parse and present Nginx logs in a user-friendly way, providing various insights and statistics.
GoAccess
GoAccess is a real-time web log analyzer that offers a terminal-based interface. To get started, install GoAccess and analyze your logs:
sudo apt-get install goaccess # On Debian/Ubuntu
sudo yum install goaccess # On CentOS/RHEL
goaccess -f /var/log/nginx/access.log
GoAccess provides interactive and HTML-based reports.
AWStats
AWStats is another popular log analyzer that generates detailed web server statistics. Install it and configure it to parse Nginx logs:
sudo apt-get install awstats # On Debian/Ubuntu
sudo yum install awstats # On CentOS/RHEL
sudo vi /etc/awstats/awstats.conf # Configure log path and other settings
/usr/lib/cgi-bin/awstats.pl -config=your-config -update
Then access the statistics via a web browser.
Using Programming Languages
You can parse Nginx logs using programming languages like Python, Ruby, or Perl, which offers more flexibility and customization.
Python
Here's a simple Python script to parse Nginx logs and count unique IP addresses:
with open('/var/log/nginx/access.log', 'r') as file:
unique_ips = set()
for line in file:
ip = line.split()[0]
unique_ips.add(ip)
print(f"Unique IP addresses: {len(unique_ips)}")
Ruby
You can achieve similar results in Ruby:
unique_ips = Set.new
File.open('/var/log/nginx/access.log', 'r') do |file|
file.each_line do |line|
ip = line.split[0]
unique_ips.add(ip)
end
end
puts "Unique IP addresses: #{unique_ips.size}"
These are just a few methods for parsing Nginx logs. Depending on your needs and preferences, you can choose the most suitable approach. Log parsing is essential for monitoring and maintaining your web server, and it can help you gain insights into your web traffic and server performance.
Log management solutions
Better Stack Logs
Better Stack is a log management solution based on ClickHouse, which allows it to be fast without compromising security or reliability. Better Stack collects data from the majority of the most popular languages, frameworks, and hosts.
It also offers advanced collaboration features, one-click filtering by context, and presence & absence monitoring, all together in a developer-centric Dark UI.
Better Stack offers one-click integration with Better Stack Uptime, an uptime monitoring and incident management tool from Better Stack. With this integration, developers can join metrics and logs, collaborate on incidents, manage on-call schedules, and create status pages from one place.
-
502 bad gateway Nginx
If you are getting the 502 bad gateway error when accessing a Nginx server, here are a few solutions: Check if Nginx is running To check if Nginx is running run the following command: systemctl sta...
Questions -
How to force or redirect to SSL in nginx?
To force or redirect all incoming traffic to SSL (HTTPS) in Nginx, you can use a server block that handles HTTP requests on port 80 and redirect them to HTTPS. Here's an example configuration: Open...
Questions -
How to generate a private key for the existing .crt file on Nginx?
Unfortunately, this is not possible. You cannot generate a private key out of an existing certificate. If it would be possible, you would be able to impersonate virtually any HTTPS webserver.
Questions -
Remove "www" and redirect to "https" with nginx
To remove the "www" from URLs and redirect all incoming traffic to HTTPS in Nginx, you can use a server block that handles both scenarios. Here's an example configuration: Open your Nginx configura...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us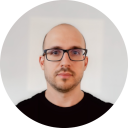
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github