How to parse Apache logs
Parsing Apache logs can be achieved through various methods and tools, allowing you to extract useful information from log files generated by the Apache web server. These logs contain a wealth of data about server requests, errors, and more. Here’s a tutorial covering several ways to parse Apache logs:
Using Command Line Tools
awk
Awk is a versatile programming language designed for text processing and pattern scanning. It operates on a line-by-line basis, allowing users to define patterns and actions to be performed when those patterns are matched. In Apache log parsing, Awk proves useful for extracting specific fields or patterns from each log entry.
The following command extracts the first and seventh fields (IP address and requested URL) from the Apache access log:
awk '{print $1, $7}' access.log
sed
Sed, short for stream editor, is a powerful text processing tool. It reads input line by line and performs specified operations based on defined patterns. Sed is handy for Apache log parsing when you need to extract and transform specific information from log entries.
This Sed command extracts IP addresses and requested URLs from the Apache access log:
sed -n 's/.*\(\b[0-9]\{1,3\}\.[0-9]\{1,3\}\.[0-9]\{1,3\}\.[0-9]\{1,3\}\b\).*"\(GET\|POST\) \(.*\) HTTP.*/\1 \3/p' access.log
Log File Analysis Tools
Webalizer
Webalizer is a robust log file analysis program designed to generate detailed statistical reports for web server activity. It supports various log formats and provides comprehensive insights into web traffic patterns over specified time intervals.
The following command generates an HTML report for a specific date range using Webalizer:
webalizer -n MyWebsite -s "January 01 2023" -e "December 31 2023" -q access.log
GoAccess
GoAccess is a real-time log analyzer that provides an interactive and dynamic view of web server logs. It supports multiple log formats, including the common Apache log formats, and allows users to visualize web traffic in real-time.
To get a live, interactive view of Apache logs with the COMBINED log format, use the following GoAccess command:
goaccess -f access.log --log-format=COMBINED
Programming Languages
Python
Python, with its rich standard library, is well-suited for log parsing tasks. The re
module facilitates regular expression matching, while the ipaddress
module aids in handling IP addresses. Python scripts provide flexibility in handling complex log parsing requirements.
The following Python script extracts IP addresses and requested URLs from the Apache access log:
import re
with open('access.log', 'r') as file:
for line in file:
match = re.search(r'(\d+\.\d+\.\d+\.\d+).*"GET (.+?) HTTP', line)
if match:
ip_address = match.group(1)
requested_url = match.group(2)
print(f'IP: {ip_address}, URL: {requested_url}')
Ruby
Ruby, known for its simplicity and readability, is adept at text processing tasks. Its concise syntax and built-in regular expression support make it suitable for log parsing. Ruby scripts can be easily adapted to extract specific information from Apache logs.
This Ruby script iterates through the Apache access log, extracting IP addresses and requested URLs:
File.open('access.log', 'r').each do |line|
match = line.match(/(\d+\.\d+\.\d+\.\d+).*"GET (.+?) HTTP/)
if match
ip_address = match[1]
requested_url = match[2]
puts "IP: #{ip_address}, URL: #{requested_url}"
end
end
C++
C++ offers high performance and low-level control, making it suitable for log parsing tasks with large datasets. Using standard C++ libraries and string manipulation functions, you can build custom parsers tailored to your specific log format.
The following C++ snippet demonstrates a basic log parser extracting IP addresses and requested URLs from an Apache access log:
#include <iostream>
#include <fstream>
#include <regex>
int main() {
std::ifstream file("access.log");
std::regex pattern(R"((\d+\.\d+\.\d+\.\d+).*"GET (.+?) HTTP)");
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::smatch match;
if (std::regex_search(line, match, pattern)) {
std::cout << "IP: " << match[1] << ", URL: " << match[2] << std::endl;
}
}
file.close();
}
return 0;
}
Log Management and Analytics Platforms
Better Stack Logs
Better Stack is a log management solution based on ClickHouse, which allows it to be fast without compromising security or reliability. Better Stack collects data from the majority of the most popular languages, frameworks, and hosts.
It also offers advanced collaboration features, one-click filtering by context, and presence & absence monitoring, all together in a developer-centric Dark UI.
Better Stack offers one-click integration with Better Stack Uptime, an uptime monitoring and incident management tool from Better Stack. With this integration, developers can join metrics and logs, collaborate on incidents, manage on-call schedules, and create status pages from one place.
Features:
- Fast log processing using ClickHouse.
- Advanced collaboration features for teams.
- One-click integration with Better Stack Uptime for metrics and incident management.
ELK Stack (Elasticsearch, Logstash, Kibana)
The ELK Stack is a powerful combination of Elasticsearch, Logstash, and Kibana. Elasticsearch is a distributed search and analytics engine, Logstash is a log pipeline tool, and Kibana is a visualization platform. Together, they provide a comprehensive solution for log management and analysis.
Features:
- Real-time log processing and indexing.
- Advanced search capabilities with a distributed architecture.
- Interactive visualizations and dashboards with Kibana.
Splunk
Splunk is a platform for log management, analytics, and security information and event management (SIEM). It collects, indexes, and correlates log data in real-time to provide insights into application performance, security, and operational intelligence.
Features:
- Powerful search and reporting capabilities.
- Machine learning-driven insights.
- Customizable dashboards and visualizations.
Graylog
Graylog is an open-source log management platform that centralizes and analyzes log data. It supports various log formats and offers a scalable architecture for handling large volumes of logs. Graylog is known for its ease of use and flexibility.
Features:
- Centralized log collection and storage.
- Streamlined search and analysis with Elasticsearch.
- Alerts and notification capabilities.
Sumo Logic
Sumo Logic is a cloud-native log management and analytics platform. It enables organizations to collect, analyze, and visualize log and machine data across the entire application stack. Sumo Logic is designed for scalability and provides real-time insights.
Features:
- Cloud-native architecture for scalability and flexibility.
- Log and event correlation for troubleshooting.
- Built-in compliance and security analytics.
LogDNA
LogDNA is a modern log management solution with a focus on simplicity and ease of use. It provides real-time log streaming, searching, and analysis, making it suitable for both small teams and large enterprises. LogDNA supports various log sources and formats.
Features:
- Real-time log streaming and search.
- Collaborative features for team-based log analysis.
- Integration with popular cloud platforms.
Considerations:
Log Format:
- Apache log formats vary. The default
CombinedLogFormat
includes various fields like IP, date, method, URL, status, etc. - Custom log formats may require specific parsing strategies.
Regular Expressions:
- Regular expressions are powerful for pattern matching and extracting specific data from log lines.
Log Rotation:
- Account for log rotation, where logs are split into multiple files (access.log, access.log.1, access.log.2, etc.).
Security and Privacy:
- Be cautious with sensitive information like IP addresses or user details when parsing logs for compliance and security reasons.
When parsing Apache logs, the approach you choose depends on your familiarity with tools, specific needs, and the level of detail required in log analysis. Different methods provide diverse ways to extract and interpret the data contained within Apache log files.
-
How can I disable TLS 1.0 and 1.1 in apache?
To disable TLS 1.0 and 1.1 in Apache, you need to modify the SSL/TLS configuration settings. This typically involves editing the Apache configuration file, such as ssl.conf or httpd.conf. Make sure...
Questions -
How do I select which Apache MPM to use?
Selecting the appropriate Apache Multi-Processing Module (MPM) depends on various factors, such as the server environment, expected traffic, and the type of workload the server will handle. Here ar...
Questions -
What does apache (busy workers, idle workers) mean?
In the context of an Apache HTTP server, the terms "busy workers" and "idle workers" pertain to the status of worker threads or processes handling incoming web requests. Busy Workers: These are the...
Questions -
How to generate a private key for the existing .crt file on Apache?
Unfortunately, this is not possible. You cannot generate a private key out of an existing certificate. If it would be possible, you would be able to impersonate virtually any HTTPS webserver.
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us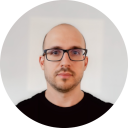
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github