How to Monitor REST APIs with Prometheus
Prometheus is an effective tool for monitoring REST APIs by collecting and analyzing metrics. By integrating Prometheus with your application or external monitoring setup, you can track key performance metrics such as response time, request count, error rates, and throughput.
1. Expose API metrics
To monitor REST APIs, you need to expose metrics in a Prometheus-compatible format. This is usually done by implementing an HTTP endpoint (e.g., /metrics
) that serves these metrics in the text-based format Prometheus understands.
Example in Python using the Prometheus Client:
Install the Prometheus client library:
pip install prometheus-client
Add metrics exposition in your application:
from prometheus_client import Counter, Histogram, start_http_server
from flask import Flask, request
app = Flask(__name__)
# Define metrics
REQUEST_COUNT = Counter('api_requests_total', 'Total API requests', ['method', 'endpoint', 'status'])
REQUEST_LATENCY = Histogram('api_request_latency_seconds', 'Latency of API requests', ['endpoint'])
@app.route('/api', methods=['GET'])
def my_api():
with REQUEST_LATENCY.labels(endpoint="/api").time(): # Track latency
status = 200
REQUEST_COUNT.labels(method='GET', endpoint='/api', status=status).inc() # Increment request counter
return {"message": "Hello, World!"}, status
if __name__ == '__main__':
start_http_server(8000) # Expose metrics on port 8000
app.run(port=5000)
- Metrics are available at
http://<host>:8000/metrics
. - Prometheus can scrape this endpoint to collect data.
2. Configure Prometheus to scrape metrics
Add a scrape job in your prometheus.yml
configuration:
scrape_configs:
- job_name: 'api-monitoring'
static_configs:
- targets: ['<your-service-host>:8000'] # Replace with your metrics endpoint
Reload Prometheus to apply the configuration.
3. Define PromQL queries for REST API monitoring
Examples of useful queries:
Total requests:
promql sum(api_requests_total)
Requests per second:
promql rate(api_requests_total[1m])
Request latency (average):
promql sum(rate(api_request_latency_seconds_sum[1m])) / sum(rate(api_request_latency_seconds_count[1m]))
Error rate:
promql sum(rate(api_requests_total{status="500"}[5m])) / sum(rate(api_requests_total[5m]))
4. Visualize metrics with Grafana
- Add Prometheus as a data source in Grafana.
- Import or create dashboards for API performance.
- Example visualizations:
- Request count over time.
- Latency heatmaps.
- Error rates.
5. Use exporters for third-party APIs
If you're monitoring external APIs, use Prometheus exporters to fetch and expose data.
Example: Blackbox Exporter
- Install Blackbox Exporter to monitor the availability and latency of REST APIs.
- Add it to Prometheus configuration:
scrape_configs:
- job_name: 'blackbox'
metrics_path: /probe
params:
module: [http_2xx] # Probe for HTTP 200 responses
static_configs:
- targets:
- https://api.example.com
relabel_configs:
- source_labels: [__address__]
target_label: __param_target
- source_labels: [__param_target]
target_label: instance
- target_label: __address__
replacement: blackbox-exporter:9115
- The Blackbox Exporter probes the target API and provides metrics like availability and response time.
By exposing metrics, configuring Prometheus, and using effective queries and visualizations, you can monitor REST APIs comprehensively with Prometheus.
-
How to Add Custom HTTP Headers in Prometheus
Here is the content with only the indentation fixed: Adding custom HTTP headers in Prometheus is useful when interacting with a secured remote endpoint, such as when scraping metrics from services ...
Questions -
How To Manage Prometheus Counters
Prometheus counters are metrics that only increase or reset to zero. They are ideal for tracking values like requests, errors, or completed tasks. Managing counters effectively ensures accurate and...
Questions -
How to install Prometheus and Grafana on Kubernetes with Helm
Installing Prometheus and Grafana on Kubernetes using Helm is a straightforward way to set up robust monitoring and visualization tools for your cluster. Helm charts simplify deployment, making con...
Questions -
Limitations of Prometheus Labels
Prometheus labels are a powerful feature used to add dimensional data to metrics. However, improper use or lack of understanding of their limitations can lead to inefficiencies, high resource consu...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us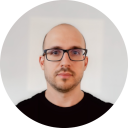
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github