How to iterate over Python dictionaries using 'for' loops?
Let’s assume the following dictionary:
my_dictionary = {
'key1': 'value1',
'key2': 'value2',
'key3': 'value3'
}
There are three main ways you can iterate over the dictionary.
Iterate over keys
If you iterate over a dictionary the same way you are iterating over a list, you will notice, that you are iterating over dictionary keys:
for k in my_dictionary:
print(k)
key1
key2
key3
This is basically the same as using the my_dictionary.keys()
as the iterable in the for loop.
You can always use the k to access the value:
for k in my_dictionary.keys():
print(my_dictionary[k])
value1
value2
value3
Iterate over values
If you want to iterate over dictionary values instead of dictionary keys, you can use the my_dictionary.values()
as iterable in the for loop:
for v in my_dictionary.values():
print(v)
value1
value2
value3
Iterate over both keys and values
To iterate over both keys and values, you can use the following syntax:
for k, v in my_dictionary.items():
print(k + ': ' + v)
key1: value1
key2: value2
key3: value3
-
Difference between static and class methods in Python?
Class method To create a class method, use the @classmethod decorator. Class methods receive the class as an implicit first argument, just like an instance method receives the instance. The class m...
Questions -
How to access the index in for loops in Python?
In python, if you are enumerating over a list using the for loop, you can access the index of the current value by using enumerate function. my_list = [1,2,3,4,5,6,7,8,9,10] for index, value in enu...
Questions -
How can I safely create a nested directory in Python?
The most common way to safely create a nested directory in Python is using the pathlib or os modules. Using pathlib You can create a nested directory in python 3.5 or later using the Path and mkdir...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us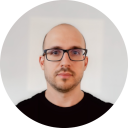
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github