How can I import a Python module dynamically given the full path?
Importing programmatically
To programmatically import a module, use importlib.import_module()
.
import importlib
itertools = importlib.import_module('itertools')
Checking if a module can be imported
If you need to find out if a module can be imported without actually doing the import, then you should use importlib.util.find_spec()
.
Note that if name
is a submodule (contains a dot), importlib.util.find_spec()
will import the parent module.
import importlib.util
import sys
# For illustrative purposes.
name = 'itertools'
if name in sys.modules:
print(f"{name!r} already in sys.modules")
elif (spec := importlib.util.find_spec(name)) is not None:
# If you chose to perform the actual import ...
module = importlib.util.module_from_spec(spec)
sys.modules[name] = module
spec.loader.exec_module(module)
print(f"{name!r} has been imported")
else:
print(f"can't find the {name!r} module")
Importing a source file directly
To import a Python source file directly, use the following recipe:
import importlib.util
import sys
# For illustrative purposes.
import tokenize
file_path = tokenize.__file__
module_name = tokenize.__name__
spec = importlib.util.spec_from_file_location(module_name, file_path)
module = importlib.util.module_from_spec(spec)
sys.modules[module_name] = module
spec.loader.exec_module(module)
Implementing lazy imports
The example below shows how to implement lazy imports:
import importlib.util
import sys
def lazy_import(name):
spec = importlib.util.find_spec(name)
loader = importlib.util.LazyLoader(spec.loader)
spec.loader = loader
module = importlib.util.module_from_spec(spec)
sys.modules[name] = module
loader.exec_module(module)
return module
lazy_typing = lazy_import("typing")
#lazy_typing is a real module object,
#but it is not loaded in memory yet.
lazy_typing.TYPE_CHECKING
-
Proper way to declare custom exceptions in modern Python?
In modern Python, the recommended way to declare custom exceptions is to create a new class that inherits from the built-in Exception class. This class should define any additional attributes or me...
Questions -
How do I remove a trailing newline in Python?
You can use the rstrip() method to remove a trailing newline from a string. Here is an example: s = "Hello, World!\n" s = s.rstrip() print(s) This will print "Hello, World!" (without the newline). ...
Questions -
How to remove an element from a list by index in Python?
To remove an element from a list by index in Python, you can use the del statement. Here is an example: a = [1, 2, 3, 4, 5] del a[2] print(a) # [1, 2, 4, 5] The del statement removes the element at...
Questions -
How to remove a key from a Python dictionary?
To remove a key from a dictionary, you can use the del statement. Here's an example: my_dict = {'a': 1, 'b': 2, 'c': 3} del my_dict['b'] print(my_dict) # Output: {'a': 1, 'c': 3} Alternatively, yo...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us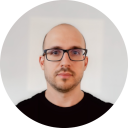
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github