How can I flush the output of the print function in Python?
In Python, you can use the flush
method of the sys.stdout
object to flush the output of the print
function. For example, you can use the following code to flush the output of the print
function:
import sys
print("Hello, World!")
sys.stdout.flush()
You can also use the file
parameter of the print
function to specify where the output should be written. For example, you can use the following code to flush the output of the print
function to a file:
import sys
with open('output.txt', 'w') as f:
print("Hello, World!", file=f)
In python 3.3 and higher, you can also use the flush
parameter of the print
function to flush the output.
print("Hello, World!", flush=True)
-
How do I print curly-brace characters in a string while using .format in Python?
To print a curly brace character in a string while using the .format() method in Python, you can use double curly braces {{ and }} to escape the curly braces. For example: print("{{example}}".forma...
Questions -
How do I print to stderr in Python?
In Python, you can use the print() function to print to stderr by passing the value file=sys.stderr as a keyword argument. For example: import sys print("This is an error message", file=sys.stderr)...
Questions -
How to print without a newline or space in Python?
To print without a new line, you need to provide one additional argument end and set its value to something other than the default \n which will break the line. You can set it to an empty character...
Questions -
How do I print colored text to the terminal in Python?
You can use ANSI escape codes to print colored text to the terminal in Python. Here is an example of how you can do this: def colored_text(color, text): colors = { "red": "\033[91m", ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us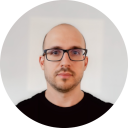
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github