How do I debug Node.js applications?
Debugging Node.js applications can be done using various tools and techniques. Here are some common approaches to debug Node.js applications:
console.log
The simplest form of debugging is using console.log
statements to print values or log messages at different points in your code. This can help you understand the flow of your program and identify potential issues.
console.log('Value of variable:', someVariable);
Node.js inspector
Node.js comes with a built-in inspector. You can start your script with the -inspect
flag and use the Chrome DevTools for debugging. The newer versions of Node.js recommend using the -inspect
flag.
node --inspect your-script.js
Then open Chrome and go to chrome://inspect
to connect to your Node.js process.
Debugger Statement
Insert the debugger
statement in your code where you want to set a breakpoint. When the script runs, it will pause at this point, allowing you to inspect variables and step through the code.
function someFunction() {
// ...
debugger;
// ...
}
Visual Studio Code (VSCode)
If you're using Visual Studio Code, it has excellent built-in support for Node.js debugging. You can set breakpoints, step through code, and inspect variables directly in the editor.
Third-Party Debugging Tools:
There are also third-party debugging tools like ndb, which provides an improved debugging experience with additional features.
npx ndb your-script.js
Remember to install ndb
globally if you want to use it without npx
.
npm install -g ndb
Choose the method that best fits your workflow and preferences. Using a combination of these tools can provide a comprehensive approach to debugging your Node.js applications.
-
Timeouts in Node.js
Learn how to implement timeouts for both incoming and outgoing requests, choose the right values, and handle timeout errors effectively
Guides -
How can I update Node.js and NPM to their latest versions?
There are several ways to update Node.js to its latest version. Here are three methods: Updating Node.js Using NPM You can use NPM to update Node.js by installing the n package, which will be used ...
Questions -
Is there a map function for objects in Node.js?
In JavaScript, the map function is typically used with arrays to transform each element of the array based on a provided callback function. If you want to achieve a similar result with objects, you...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us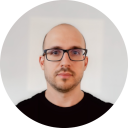
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github