How to check if an object has an attribute in Python?
To check if an object has an attribute in Python, you can use the hasattr
function. This function takes two arguments: the object and the attribute name as a string. It returns True
if the object has the attribute, and False
if it does not.
For example:
class MyClass:
def __init__(self):
self.attribute = 'attribute'
obj = MyClass()
hasattr(obj, 'attribute') # returns True
hasattr(obj, 'other_attribute') # returns False
You can also use the getattr
function to check if an object has an attribute, but hasattr
is generally more convenient.
class MyClass:
def __init__(self):
self.attribute = 'attribute'
obj = MyClass()
getattr(obj, 'attribute', None) # returns 'attribute'
getattr(obj, 'other_attribute', None) # returns None
Note that hasattr
and getattr
will only work for attributes that are defined on the object itself, or on its class or any of its superclasses. They will not work for attributes that are stored in the object's __dict__
attribute, unless the attribute is defined in the class's __slots__
attribute.
-
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions -
Finding the index of an item in a Python list?
To find an index of the first occurrence of an element in a given list, you can use index method of List class with the element passed as an argument. The syntax is the following: my_list = [1,2,0,...
Questions -
Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8. Here is an ex...
Questions -
How do I get the last element of a list in Python?
To get the last element of a list in Python, you can use the negative indexing feature of the list data type. For example: mylist = [1, 2, 3, 4, 5] lastelement = mylist[-1] # lastelement will be 5...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us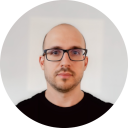
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github