How is an HTTP POST request made in node.js?
In Node.js, you can make an HTTP POST request using the http
or https
modules that come with Node.js, or you can use a more user-friendly library like axios
or node-fetch
. Here, I'll provide examples for both the core modules and the axios
library.
Using http
Module (Core Module)
const http = require('http');
// Example POST data (can be a JSON object or any data)
const postData = JSON.stringify({
key1: 'value1',
key2: 'value2'
});
// Options for the HTTP request
const options = {
hostname: 'example.com',
port: 80,
path: '/path/to/api',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Content-Length': Buffer.byteLength(postData)
}
};
// Create the HTTP request
const req = http.request(options, (res) => {
let responseData = '';
// A chunk of data has been received.
res.on('data', (chunk) => {
responseData += chunk;
});
// The whole response has been received.
res.on('end', () => {
console.log('Response:', responseData);
});
});
// Handle errors
req.on('error', (error) => {
console.error('Error:', error.message);
});
// Send the POST data
req.write(postData);
req.end();
Using axios
Library
First, you need to install axios
using:
npm install axios
Then, you can use it in your Node.js script:
const axios = require('axios');
// Example POST data (can be a JSON object or any data)
const postData = {
key1: 'value1',
key2: 'value2'
};
// Make a POST request using axios
axios.post('<https://example.com/path/to/api>', postData)
.then((response) => {
console.log('Response:', response.data);
})
.catch((error) => {
console.error('Error:', error.message);
});
Using a library like axios
simplifies the process and provides additional features like automatic JSON parsing and promise-based syntax.
Choose the approach that fits your requirements and coding style.
-
How can I get the full object in Node.js's console.log(), rather than '[Object]'?
By default, console.log() in Node.js will display [Object] when trying to log an object. If you want to see the full contents of an object, you can use util.inspect() from the util module, which is...
Questions -
How can I update Node.js and NPM to their latest versions?
There are several ways to update Node.js to its latest version. Here are three methods: Updating Node.js Using NPM You can use NPM to update Node.js by installing the n package, which will be used ...
Questions -
How to get `GET` variables in Express.js on Node.js?
In Express.js, you can access GET (query string) variables using the req.query object. The req.query object contains key-value pairs of the query string parameters in a GET request. Here's an examp...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us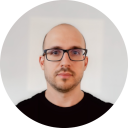
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github