How Do You Pass Parameters to Fixtures in Pytest?
In pytest, passing parameters to fixtures instead of directly to test functions can enhance code reusability and simplify test setups.
For example, if you're testing file handling, you might pass file paths directly to your test functions. This approach requires you to create an instance of the FileReader
class in each test, as shown:
import pytest
class FileReader:
def __init__(self, file_path):
self.file_path = file_path
def read_content(self):
with open(self.file_path, 'r') as file:
return file.read()
class TestFileReader:
@pytest.mark.parametrize('file_path', ['file1.txt', 'file2.txt'])
def test_read_content(self, file_path):
reader = FileReader(file_path)
content = reader.read_content()
assert content == "Expected content"
In this example, each test function creates a FileReader
instance.
However, a more elegant solution involves using pytest fixtures with indirect=True
. This approach allows you to create a fixture that handles the initialization of the FileReader
object, which can then be injected into your tests:
import pytest
class FileReader:
def __init__(self, file_path):
self.file_path = file_path
def read_content(self):
with open(self.file_path, 'r') as file:
return file.read()
@pytest.fixture
def file_reader(request):
return FileReader(request.param)
class TestFileReader:
@pytest.mark.parametrize('file_reader', ['file1.txt', 'file2.txt'], indirect=True)
def test_read_content(self, file_reader):
content = file_reader.read_content()
assert content == "Expected content"
Here, the file_reader
fixture constructs the FileReader
instance. The indirect=True
parameter in @pytest.mark.parametrize
instructs pytest to pass the parameters to the fixture rather than directly to the test function. This method centralizes object creation and allows the tests to focus on verifying behaviour, which is especially beneficial for complex setups or when reusing setups across multiple tests.
-
How to Disable a Test Using Pytest?
If you need to disable a specific test when running your test suite with pytest, use the pytest skip decorator. Suppose you have the following tests in your test suite: import pytest def test_addit...
Questions -
How to Test a Single File Under Pytest
To run a single test file with pytest, use the command pytest followed by the file path: pytest tests/test_file.py To execute a specific test within that file, append :: and the test name to the fi...
Questions -
How to Skip Directories With Pytest?
You can instruct Pytest to exclude specific directories from testing with the --ignore option. To exclude a single directory, execute: pytest --ignore=somedirectory To exclude multiple directories ...
Questions -
How to Assert Almost Equal in Pytest?
To assert almost equal in Pytest, use the approx() method. This is useful for floating-point comparisons that may involve small rounding errors. Here's how to use it: import pytest def testapproxeq...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us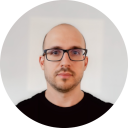
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github