How Can I Log From Python to Syslog With Either Sysloghandler or Syslog on Mac Os X And Debian (7)
Logging from Python to syslog on both macOS and Debian can be done using the SysLogHandler
class from the logging
module, which is available in the Python standard library. This handler sends log messages to the syslog service. Here's a step-by-step guide on how to set this up:
1. Using SysLogHandler
in Python
The SysLogHandler
class is part of the logging.handlers
module. It allows Python applications to send log messages to the system’s syslog service.
Basic Usage
Here’s a basic example of how to configure Python’s logging to send messages to syslog:
import logging
import logging.handlers
# Create a logger
logger = logging.getLogger('myapp')
logger.setLevel(logging.DEBUG)
# Create a SysLogHandler
syslog_handler = logging.handlers.SysLogHandler(address='/dev/log') # For Unix-based systems
# Optional: Set a formatter
formatter = logging.Formatter('%(asctime)s %(name)s %(levelname)s: %(message)s')
syslog_handler.setFormatter(formatter)
# Add the SysLogHandler to the logger
logger.addHandler(syslog_handler)
# Log a message
logger.info('This is a test log message')
Configuration for macOS and Debian
- macOS: On macOS, the syslog address is typically
/var/run/syslog
or/dev/log
. - Debian: On Debian, the syslog address is usually
/dev/log
.
You can adjust the address
parameter in SysLogHandler
based on your system:
# For macOS
syslog_handler = logging.handlers.SysLogHandler(address='/var/run/syslog')
# For Debian
syslog_handler = logging.handlers.SysLogHandler(address='/dev/log')
2. Ensuring Syslog is Running
Ensure that the syslog service is running on your system. On Debian, you typically have rsyslog
running, and on macOS, the built-in syslog
service is used.
Check Syslog Status
Debian:
sudo systemctl status rsyslog
macOS:
The
syslog
service should be running by default. You can check its status using:sudo syslog -c
3. Verify Logging
After running your Python script, verify that the log message appears in the syslog:
Debian: Check the syslog file (usually
/var/log/syslog
or/var/log/messages
):tail -f /var/log/syslog
macOS: Check the syslog using
Console.app
or by viewing logs in the terminal:log show --predicate 'process == "myapp"' --info
4. Advanced Configuration
You can configure additional settings such as log levels or different logging formats by modifying the logger configuration.
Example with Advanced Configuration
import logging
import logging.handlers
# Create a logger
logger = logging.getLogger('myapp')
logger.setLevel(logging.DEBUG)
# Create a SysLogHandler
syslog_handler = logging.handlers.SysLogHandler(address='/dev/log') # Adjust address based on your OS
# Set a formatter
formatter = logging.Formatter('%(asctime)s %(name)s %(levelname)s: %(message)s')
syslog_handler.setFormatter(formatter)
# Add the SysLogHandler to the logger
logger.addHandler(syslog_handler)
# Log messages of different severity levels
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
This setup should work on both macOS and Debian systems for logging to syslog from Python. If you have specific requirements or encounter issues, let me know!
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us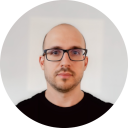
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github