Trusting All Certificates Using Httpclient Over Https
Trusting all certificates when making HTTPS requests using HttpClient
is generally not recommended due to significant security risks. However, for development or testing purposes, you might need to bypass SSL certificate validation. Here’s how you can do this for various platforms and languages:
1. Python: Using requests
with urllib3
For Python's requests
library, you can bypass SSL verification by configuring a custom adapter or by setting the verify
parameter to False
.
Option 1: Disable SSL Verification (Not Recommended for Production)
import requests
# Bypass SSL verification
response = requests.get('<https://example.com>', verify=False)
print(response.text)
Option 2: Use a Custom Adapter
You can create a custom adapter to ignore SSL verification:
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.poolmanager import PoolManager
import ssl
class SSLAdapter(HTTPAdapter):
def init_poolmanager(self, *args, **kwargs):
kwargs['ssl_context'] = ssl._create_unverified_context()
return super().init_poolmanager(*args, **kwargs)
session = requests.Session()
session.mount('https://', SSLAdapter())
response = session.get('<https://example.com>')
print(response.text)
2. Java: Using HttpClient
In Java, you can configure HttpClient
to trust all certificates. This involves setting up a custom TrustManager
that accepts all certificates.
Option 1: Trust All Certificates (For Development/Testing)
import javax.net.ssl.*;
import java.security.cert.X509Certificate;
import java.security.KeyStore;
import java.net.HttpURLConnection;
import java.net.URL;
public class TrustAllCertificates {
public static void main(String[] args) throws Exception {
// Create a TrustManager that trusts all certificates
TrustManager[] trustAll = new TrustManager[]{
new X509TrustManager() {
public X509Certificate[] getAcceptedIssuers() {
return null;
}
public void checkClientTrusted(X509Certificate[] certs, String authType) {
}
public void checkServerTrusted(X509Certificate[] certs, String authType) {
}
}
};
// Install the all-trusting trust manager
SSLContext sc = SSLContext.getInstance("SSL");
sc.init(null, trustAll, new java.security.SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory());
// Create an all-trusting host verifier
HostnameVerifier allHostsValid = new HostnameVerifier() {
public boolean verify(String hostname, SSLSession session) {
return true;
}
};
// Install the all-trusting host verifier
HttpsURLConnection.setDefaultHostnameVerifier(allHostsValid);
// Make a request
URL url = new URL("<https://example.com>");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.connect();
// Read the response
System.out.println("Response Code: " + connection.getResponseCode());
}
}
3. JavaScript: Using axios
with Node.js
For Node.js applications using axios
, you can configure it to ignore SSL certificate validation errors.
Option 1: Bypass SSL Verification
const axios = require('axios');
const https = require('https');
// Create an instance of axios with HTTPS agent configured to ignore SSL errors
const instance = axios.create({
httpsAgent: new https.Agent({ rejectUnauthorized: false })
});
instance.get('<https://example.com>')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error:', error);
});
Security Considerations
- Risk: Trusting all certificates or disabling SSL verification exposes your application to various security risks, including man-in-the-middle (MITM) attacks and data breaches.
- Use Case: Such configurations should only be used in development or testing environments where security is not a concern.
- Production: For production environments, always use valid, trusted SSL certificates and ensure proper SSL/TLS verification is in place.
Summary
Bypassing SSL certificate verification can be done in various programming environments for development or testing purposes. However, in production, it is crucial to use valid certificates and ensure proper SSL/TLS security measures are in place to protect against potential security threats.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us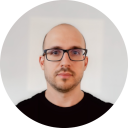
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github