How to Create Static Methods in Python?
In Python, a static method is a method that belongs to a class but does not operate on instances of that class. Unlike instance methods, static methods do not have access to the instance (self
) or class (cls
) variables. They are defined using the @staticmethod
decorator.
Here's how you can define and use static methods in Python:
class MyClass:
# Static method definition using @staticmethod decorator
@staticmethod
def static_method():
print("This is a static method")
# Calling the static method using the class name
MyClass.static_method()
In this example:
static_method()
is defined within theMyClass
class and decorated with@staticmethod
.- Static methods do not require a reference to an instance (e.g.,
self
) or the class itself (e.g.,cls
) as their first parameter. - You can call a static method using the class name, as shown with
MyClass.static_method()
.
Static methods are often used for utility functions that are related to the class but do not depend on specific instance data. They can be called without creating an instance of the class.
It's important to note that while static methods are associated with a class, they cannot access or modify class or instance variables directly. They are primarily used for organizing code within a class namespace and for providing utility functions.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us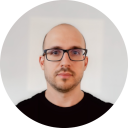
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github