module.exports vs exports in Node.js
In Node.js, both module.exports
and exports
are used to define the exports of a module, but there are differences in how they can be used.
module.exports
module.exports
is the actual object that is returned when you require a module in another file.
When you assign a value directly to module.exports
, you replace the entire exports object with your new value.
You can assign any type of value to module.exports
, including functions, objects, or primitive values.
// file: myModule.js
// Assigning a function to module.exports
module.exports = function() {
console.log('Hello from myModule!');
};
exports
exports
is a shorthand reference to module.exports
.
You can use exports
to add properties or methods to the exports object, but you cannot directly assign a new value to exports
.
If you try to assign a new value directly to exports
, it will break the reference between exports
and module.exports
.
// file: myModule.js
// Adding a property to exports
exports.myFunction = function() {
console.log('Hello from myModule!');
};
However, you should avoid directly reassigning exports
if you want to maintain the reference to module.exports
. If you reassign exports
, it will no longer point to module.exports
, and your module might not work as expected.
When to use which:
If you are exporting a single object, function, or value, you can use either module.exports
or exports
.
If you are extending exports with additional properties or methods, use exports
.
If you need to replace the entire exports object, use module.exports
.
// Preferable usage when extending exports
exports.myFunction = function() {
console.log('Hello from myModule!');
};
exports.anotherFunction = function() {
console.log('Another function!');
};
// If you need to replace the entire exports object
module.exports = {
myFunction: function() {
console.log('Hello from myModule!');
},
anotherFunction: function() {
console.log('Another function!');
}
};
In most cases, developers use module.exports
when they want to replace the entire exports object and use exports
for extending the exports with additional properties or methods. It's essential to be consistent in your usage to avoid potential confusion.
-
Is there a map function for objects in Node.js?
In JavaScript, the map function is typically used with arrays to transform each element of the array based on a provided callback function. If you want to achieve a similar result with objects, you...
Questions -
How to read environment variables in Node.js
In Node.js, you can read environmental variables using the process.env object. This object provides access to the user environment, including environment variables. Here's a simple example of how y...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us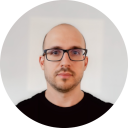
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github