How to write to files in Node.js?
In Node.js, you can use the fs
(file system) module to read from and write to files. Here's a basic example of how to write and read files in Node.js using callbacks:
const fs = require('fs');
// Write to the file
fs.writeFile('filePath', 'dataToWrite', (err) => {
if (err) {
console.error('Error writing to file:', err);
} else {
console.log('Data has been written to the file successfully!');
}
});
// Read from the file
fs.readFile('filePath', 'utf8', (err, data) => {
if (err) {
console.error('Error reading from file:', err);
} else {
console.log('Data read from file:', data);
}
});
In the examples above:
writeFile
is used to write data to a file. It takes the file path, the data to be written, and a callback function that will be called when the write operation is complete.readFile
is used to read data from a file. It takes the file path, the encoding (in this case, 'utf8' for text files), and a callback function that will be called with the data read from the file.
Remember that these operations are asynchronous, so using callbacks or promises is essential to handle the results properly.
Additionally, starting from Node.js version 10, you can use the promisify
utility from the util
module to convert these functions into promises, making it easier to work with async/await syntax. Example can be seen below:
const fs = require('fs');
const util = require('util');
// Promisify the fs functions to use async/await
const writeFileAsync = util.promisify(fs.writeFile);
const readFileAsync = util.promisify(fs.readFile);
async function writeToFile() {
const dataToWrite = 'Hello, this is some data to write to a file!';
const filePath = 'example.txt';
try {
await writeFileAsync(filePath, dataToWrite);
console.log('Data has been written to the file successfully!');
} catch (err) {
console.error('Error writing to file:', err);
}
}
async function readFromFile() {
const filePath = 'example.txt';
try {
const data = await readFileAsync(filePath, 'utf8');
console.log('Data read from file:', data);
} catch (err) {
console.error('Error reading from file:', err);
}
}
// Example usage
writeToFile();
readFromFile();
In this example:
- The
writeFileAsync
andreadFileAsync
functions are created using thepromisify
utility from theutil
module. - The
writeToFile
andreadFromFile
functions useasync/await
syntax for better readability. - Error handling is done using try-catch blocks, making it easier to handle both successful and failed operations.
This approach simplifies the code and makes it more readable, especially when dealing with multiple asynchronous file operations.
-
Job Scheduling in Node.js with BullMQ
This is a comprehensive guide for anyone looking to implement task scheduling with BullMQ in a Node.js application
Guides -
How can I update Node.js and NPM to their latest versions?
There are several ways to update Node.js to its latest version. Here are three methods: Updating Node.js Using NPM You can use NPM to update Node.js by installing the n package, which will be used ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us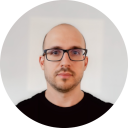
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github