How to Remove File in node.js
In Node.js, you can remove (delete) a file using the built-in fs
(File System) module. The fs
module provides the unlink
function for this purpose. Here's an example:
const fs = require('fs');
const filePath = '/path/to/your/file.txt'; // Replace with the actual path to your file
// Remove the file
fs.unlink(filePath, (err) => {
if (err) {
console.error(`Error removing file: ${err}`);
return;
}
console.log(`File ${filePath} has been successfully removed.`);
});
In this example:
- Replace
'/path/to/your/file.txt'
with the actual path to the file you want to remove. - The
fs.unlink
function is used to delete the specified file. It takes the file path and a callback function as arguments. - The callback function is called once the file has been removed. If there's an error, it will be passed to the callback.
Make sure to handle errors appropriately, as attempting to remove a non-existent file or a file in use could result in an error.
Note: Deleting files is a destructive operation, so use it with caution. Always check for errors and consider adding proper error handling based on your application's requirements.
-
Job Scheduling in Node.js with Agenda
This article provides a comprehensive guide for anyone looking to implement effective task scheduling in a Node.js application
Guides -
Comparing Node.js Testing Libraries
This guide presents a comparison of top 9 testing libraries for Node.js to help you decide which one to use in your next project
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us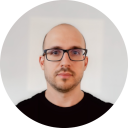
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github