How to exit in Node.js
Exiting a Node.js application is simple and can be done using the process.exit()
method. This method exits from the current Node.js process and takes an exit code, which is an integer. The exit code can be either 0 or 1. 0 means end the process without any kind of failure and 1 means end the process with some failure.
Here’s how you can use the process.exit()
method:
if (someConditionNotMet()) {
console.log('Usage: node <filename>');
process.exit(1);
}
In the above example, if someConditionNotMet()
returns true
, the message Usage: node <filename>
is printed to the console and the process exits with an exit code of 1.
It’s important to note that calling process.exit()
will force the process to exit as quickly as possible even if there are still asynchronous operations pending that have not yet completed fully, including I/O operations to process.stdout
and process.stderr
In most situations, it is not actually necessary to call process.exit()
explicitly. The Node.js process will exit on its own if there is no additional work pending in the event loop.
Here are some other ways to exit a Node.js application:
- Throwing an Error: You can throw an error to exit a Node.js application. This method is useful when you want to exit the application with a specific error message.
throw new Error('Something went wrong');
- Using the
process.kill()
Method: You can use theprocess.kill()
method to terminate a Node.js process. This method sends a signal to the process to terminate it. The signal can be eitherSIGTERM
orSIGKILL
.
process.kill(process.pid, 'SIGTERM');
-
How to run Cron jobs in Node.js?
To run Cron jobs in Node.js, you can use a Node.js package called node-cron. Here are the steps to create and schedule a Node.js script using node-cron: Install node-cron You can install node-cron ...
Questions -
How do I pass command line arguments to a Node.js program and receive them?
In Node.js, you can pass command line arguments to your script the same as you would for any other command line application. Simply type your arguments after the script path separated with a space ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us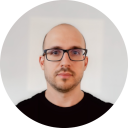
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github