How to Fix Error: Request Entity Too Large
The "Error: request entity too large" typically occurs when the payload of a HTTP request exceeds the size limit set by the server. This can happen in various contexts, such as when handling file uploads, form submissions, or any other request that includes a large amount of data.
To fix this error, you can take different approaches depending on the context:
Express.js (Handling Form Data)
If you're using Express.js to handle form data, you can adjust the body-parser
middleware to increase the request size limit.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
// Increase the request size limit (adjust the limit based on your needs)
app.use(bodyParser.json({ limit: '50mb' }));
app.use(bodyParser.urlencoded({ limit: '50mb', extended: true }));
// Your other Express middleware and routes...
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Express.js (Handling File Uploads)
If you're dealing with file uploads, you may need to adjust the limits for both body-parser
and multer
middleware.
const express = require('express');
const bodyParser = require('body-parser');
const multer = require('multer');
const app = express();
// Increase the request size limit for body-parser
app.use(bodyParser.json({ limit: '50mb' }));
app.use(bodyParser.urlencoded({ limit: '50mb', extended: true }));
// Increase the request size limit for multer
const upload = multer({ limits: { fileSize: 50 * 1024 * 1024 } }); // 50MB limit
app.post('/upload', upload.single('file'), (req, res) => {
// Handle the uploaded file
res.status(200).json({ message: 'File uploaded successfully' });
});
// Your other Express middleware and routes...
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Native HTTP Server (Without Express)
If you're using the native http
module to create an HTTP server, you can handle request data directly. Here's a simple example:
const http = require('http');
const server = http.createServer((req, res) => {
let data = '';
req.on('data', (chunk) => {
// Accumulate the request data
data += chunk;
// Check if the data size exceeds the limit
if (data.length > 50 * 1024 * 1024) {
res.writeHead(413, 'Request Entity Too Large', { 'Content-Type': 'text/plain' });
res.end('Request entity too large');
req.connection.destroy(); // Terminate the request
}
});
req.on('end', () => {
// Process the accumulated data
// ...
// Send a response
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('OK');
});
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
Adjust the limits and error handling based on your specific use case and requirements. Always be cautious when increasing request size limits, as it may have implications for your server's performance and security.
-
Execute a Command Line Binary with node.js
You can execute a command line binary in Node.js using the child_process module. Here's a simple example: const { exec } = require('child_process'); const binaryPath = '/path/to/your/binary'; // Re...
Questions -
How to reduce size of node_modules folder?
Reducing the size of the node_modules folder in a Node.js project can be important, especially when deploying applications or managing version control. Here are several strategies you can use to mi...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us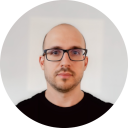
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github