How to Use All the Cores on Multi-Core Machines in node.js
Node.js is designed to be single-threaded, but you can take advantage of multi-core machines by utilizing the cluster
module or by creating separate Node.js processes manually.
Using the cluster
Module
The cluster
module allows you to create child processes (workers) that share the same port, enabling load balancing across CPU cores. Here's a basic example:
const cluster = require('cluster');
const os = require('os');
if (cluster.isMaster) {
// Fork workers
for (let i = 0; i < os.cpus().length; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
});
} else {
// Your application logic here
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello from worker ' + cluster.worker.id);
});
app.listen(3000, () => {
console.log(`Worker ${cluster.worker.id} running`);
});
}
In this example, the master process forks child processes, each running a worker. Each worker is a separate instance of your application, and they can share the load.
Using Multiple Processes Manually
Another approach is to create multiple Node.js processes manually and distribute the workload. This can be done using a process manager like pm2
or by using tools like child_process
to spawn multiple Node.js processes. Here's a simplified example:
const { fork } = require('child_process');
const os = require('os');
// Fork processes
for (let i = 0; i < os.cpus().length; i++) {
const worker = fork('worker.js');
console.log(`Forked worker ${worker.pid}`);
}
// worker.js
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello from worker ' + process.pid);
});
app.listen(3000, () => {
console.log(`Worker ${process.pid} running`);
});
This example manually forks multiple Node.js processes, each running the same worker.js
application.
Choose the method that best fits your application and deployment environment. Note that effective load balancing may require additional considerations, and the choice between these methods depends on your specific use case.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us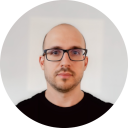
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github